torch写一个loss
时间: 2023-08-19 18:50:44 浏览: 41
当使用 PyTorch 编写损失函数时,你可以创建一个继承自 `torch.nn.Module` 的自定义类来定义你的损失函数。下面是一个示例,展示如何编写一个简单的均方误差(MSE)损失函数:
```python
import torch
import torch.nn as nn
class CustomLoss(nn.Module):
def __init__(self):
super(CustomLoss, self).__init__()
def forward(self, predicted, target):
loss = torch.mean((predicted - target) ** 2)
return loss
```
在上面的示例中,我们创建了一个名为 `CustomLoss` 的自定义损失函数类。在 `forward` 方法中,我们计算了预测值 `predicted` 与目标值 `target` 之间的均方误差,并返回该损失值。
你可以根据需要自定义其他类型的损失函数,只需在 `forward` 方法中实现相应的计算逻辑即可。
相关问题
写一个test loss代码
要写一个 Test Loss 的代码,需要先有一个模型和测试数据集。以下是一个简单的示例代码,可以计算出在测试数据集上的平均损失值:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader
# 定义模型
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# 加载测试数据集
test_data = [(torch.randn(10), torch.randn(1)) for _ in range(100)]
test_loader = DataLoader(test_data, batch_size=10)
# 加载模型
model = MyModel()
model.load_state_dict(torch.load('model.pth'))
# 定义损失函数
criterion = nn.MSELoss()
# 计算测试集上的平均损失
test_loss = 0.0
with torch.no_grad():
model.eval()
for inputs, targets in test_loader:
outputs = model(inputs)
loss = criterion(outputs, targets)
test_loss += loss.item() * inputs.size(0)
test_loss /= len(test_data)
print(f"Test Loss: {test_loss:.4f}")
```
在这个示例代码中,我们定义了一个简单的模型 `MyModel`,并加载了一个测试数据集 `test_data`。在计算测试集上的损失时,我们使用了 PyTorch 中的 `DataLoader` 来批量加载数据。我们使用了 `nn.MSELoss()` 作为损失函数,计算每个批次的损失,最后求平均值得到测试集上的平均损失值。
帮我写一个focal loss
好的,下面是一个简单的 PyTorch 实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class FocalLoss(nn.Module):
def __init__(self, gamma=2, alpha=None, reduction='mean'):
super(FocalLoss, self).__init__()
self.gamma = gamma
self.alpha = alpha
self.reduction = reduction
def forward(self, inputs, targets):
ce_loss = F.cross_entropy(inputs, targets, reduction='none')
pt = torch.exp(-ce_loss)
focal_loss = (1 - pt) ** self.gamma * ce_loss
if self.alpha is not None:
alpha = self.alpha[targets]
focal_loss = alpha * focal_loss
if self.reduction == 'mean':
return focal_loss.mean()
elif self.reduction == 'sum':
return focal_loss.sum()
else:
return focal_loss
```
其中,gamma 表示 Focal Loss 中的一个超参数,alpha 可以用来调整不同类别的权重,reduction 表示损失函数的计算方式(mean 或 sum)。在 forward 函数中,首先计算 cross-entropy loss,然后计算 focal loss,最后根据 alpha 和 reduction 进行处理并返回。
相关推荐
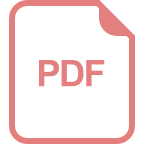
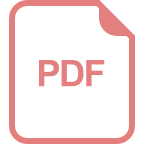
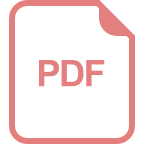












