for k, col in zip(range(n_clusters_), colors): class_members = labels == k cluster_center = data_points[cluster_centers_indices[k]] plt.plot(data_points[class_members, 0], data_points[class_members, 1], 'w', markerfacecolor=col, marker='.') plt.plot(cluster_center[0], cluster_center[1], 'o', markerfacecolor=col, markersize=10) 这段代码什么意思
时间: 2023-05-28 13:01:25 浏览: 153
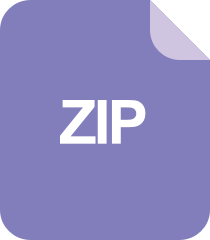
Clustering_K_means_clustering.zip_This Is It
这段代码用于将通过聚类算法分出来的数据点和聚类中心画在二维平面上。具体解释如下:
- 对于每一个聚类k,取出属于该聚类的数据点(class_members),用颜色col标记并以小圆点(marker)画出来。
- 取出聚类中心坐标(cluster_center),用颜色col标记并以大圆点(marker)画出来。大小为10。
- 通过zip函数将k和colors串联起来,循环遍历所有聚类并分别画图。其中n_clusters_表示总聚类数。
阅读全文
相关推荐
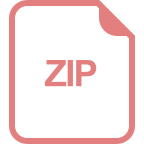















