def create_LSTM_model(): # instantiate the model model = Sequential() model.add(Input(shape=(X_train.shape[1], X_train.shape[2]))) X_train = X_train.reshape((X_train.shape[0], n_steps, 1, n_length, n_features)) # cnn1d Layers model.add(ConvLSTM2D(filters=64, kernel_size=(1,3), activation='relu', input_shape=(n_steps, 1, n_length, n_features))) model.add(Flatten()) model.add(RepeatVector(n_outputs)) model.add(MaxPooling1D()) # 添加lstm层 model.add(LSTM(64, activation = 'relu', return_sequences=True)) model.add(Dropout(0.5)) #添加注意力层 model.add(LSTM(64, activation = 'relu', return_sequences=False)) # 添加dropout model.add(Dropout(0.5)) model.add(Dense(128)) # 输出层 model.add(Dense(1, name='Output')) # 编译模型 model.compile(optimizer='adam', loss='mse', metrics=['mae']) return model # lstm network model = create_LSTM_model() # summary print(model.summary())修改该代码,解决UnboundLocalError: local variable 'X_train' referenced before assignment问题
时间: 2023-12-05 19:04:00 浏览: 174
在函数中,我们应该在使用变量 `X_train` 之前先对其进行赋值。因此,我们需要将 `X_train = X_train.reshape((X_train.shape[0], n_steps, 1, n_length, n_features))` 这一行代码的位置提前,放在 `model.add(Input(shape=(X_train.shape[1], X_train.shape[2])))` 的前面即可解决问题。修改后的代码如下:
```
def create_LSTM_model():
# instantiate the model
model = Sequential()
X_train = X_train.reshape((X_train.shape[0], n_steps, 1, n_length, n_features))
model.add(Input(shape=(X_train.shape[1], X_train.shape[2])))
# cnn1d Layers
model.add(ConvLSTM2D(filters=64, kernel_size=(1,3), activation='relu',
input_shape=(n_steps, 1, n_length, n_features)))
model.add(Flatten())
model.add(RepeatVector(n_outputs))
model.add(MaxPooling1D())
# 添加lstm层
model.add(LSTM(64, activation='relu', return_sequences=True))
model.add(Dropout(0.5))
#添加注意力层
model.add(LSTM(64, activation='relu', return_sequences=False))
# 添加dropout
model.add(Dropout(0.5))
model.add(Dense(128))
# 输出层
model.add(Dense(1, name='Output'))
# 编译模型
model.compile(optimizer='adam', loss='mse', metrics=['mae'])
return model
# lstm network
model = create_LSTM_model()
# summary
print(model.summary())
```
阅读全文
相关推荐
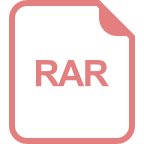







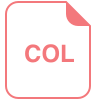
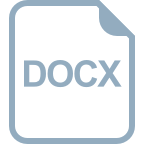
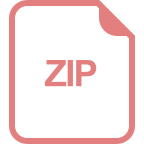