最小重量机器设计问题,用c++
时间: 2023-12-03 11:45:23 浏览: 35
最小重量机器设计问题是指在给定的约束条件下,设计出一台重量最小的机器。这可以看作是一个优化问题,常用的求解方法有线性规划、动态规划、贪心算法等。
以下是一个使用线性规划求解最小重量机器设计问题的C++代码示例:
```c++
#include <iostream>
#include <vector>
#include <cmath>
#include <ilcplex/ilocplex.h> // 引入CPLEX库头文件
using namespace std;
// 定义机器结构体
struct Machine {
double x, y, z, density; // 机器的三个维度和密度
};
int main() {
int n; // 机器数量
cin >> n;
vector<Machine> machines(n); // 存储所有机器的向量
for (int i = 0; i < n; i++) {
cin >> machines[i].x >> machines[i].y >> machines[i].z >> machines[i].density;
}
IloEnv env; // 创建CPLEX环境
try {
IloModel model(env); // 创建CPLEX模型
IloNumVarArray x(env, n, 0.0, IloInfinity, ILOFLOAT); // 定义x变量
IloNumVarArray y(env, n, 0.0, IloInfinity, ILOFLOAT); // 定义y变量
IloNumVarArray z(env, n, 0.0, IloInfinity, ILOFLOAT); // 定义z变量
// 添加约束条件:机器的总重量不能超过一定值
IloExpr weight(env);
for (int i = 0; i < n; i++) {
weight += machines[i].density * x[i] * y[i] * z[i];
}
model.add(weight <= 10000);
weight.end();
// 添加目标函数:最小化机器的总重量
IloExpr obj(env);
for (int i = 0; i < n; i++) {
obj += machines[i].density * x[i] * y[i] * z[i];
}
model.add(IloMinimize(env, obj));
obj.end();
// 解决优化问题
IloCplex cplex(model);
cplex.solve();
// 输出结果
cout << "Minimum weight: " << cplex.getObjValue() << endl;
for (int i = 0; i < n; i++) {
cout << "Machine " << i + 1 << ": " << x[i].getValue() << ", " << y[i].getValue() << ", " << z[i].getValue() << endl;
}
} catch (IloException& e) {
cerr << "Error: " << e.getMessage() << endl;
} catch (...) {
cerr << "Unknown exception caught!" << endl;
}
env.end(); // 释放CPLEX环境
return 0;
}
```
这个示例代码使用了CPLEX库来求解线性规划问题,需要在编译时链接CPLEX库。为了简化代码,省略了头文件和链接库的部分。如果需要运行这个程序,请根据自己的环境配置相关设置。
相关推荐
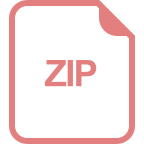














