编写java web 学生选课系统代码
时间: 2023-10-12 16:04:44 浏览: 42
由于该项目比较复杂,无法在短时间内完成。以下是一个简单的Java Web学生选课系统的代码示例:
Student.java:
```java
public class Student {
private int id;
private String name;
private List<Course> courses;
public Student(int id, String name) {
this.id = id;
this.name = name;
this.courses = new ArrayList<>();
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public List<Course> getCourses() {
return courses;
}
public void addCourse(Course course) {
courses.add(course);
}
public void removeCourse(Course course) {
courses.remove(course);
}
}
```
Course.java:
```java
public class Course {
private int id;
private String name;
private int credit;
private List<Student> students;
public Course(int id, String name, int credit) {
this.id = id;
this.name = name;
this.credit = credit;
this.students = new ArrayList<>();
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getCredit() {
return credit;
}
public List<Student> getStudents() {
return students;
}
public void addStudent(Student student) {
students.add(student);
}
public void removeStudent(Student student) {
students.remove(student);
}
}
```
StudentDAO.java:
```java
public class StudentDAO {
private List<Student> students;
public StudentDAO() {
this.students = new ArrayList<>();
}
public List<Student> getAllStudents() {
return students;
}
public Student getStudentById(int id) {
for (Student student : students) {
if (student.getId() == id) {
return student;
}
}
return null;
}
public void addStudent(Student student) {
students.add(student);
}
public void removeStudent(Student student) {
students.remove(student);
}
}
```
CourseDAO.java:
```java
public class CourseDAO {
private List<Course> courses;
public CourseDAO() {
this.courses = new ArrayList<>();
}
public List<Course> getAllCourses() {
return courses;
}
public Course getCourseById(int id) {
for (Course course : courses) {
if (course.getId() == id) {
return course;
}
}
return null;
}
public void addCourse(Course course) {
courses.add(course);
}
public void removeCourse(Course course) {
courses.remove(course);
}
}
```
MainController.java:
```java
@WebServlet("/")
public class MainController extends HttpServlet {
private StudentDAO studentDAO;
private CourseDAO courseDAO;
@Override
public void init() throws ServletException {
super.init();
studentDAO = new StudentDAO();
courseDAO = new CourseDAO();
Course course1 = new Course(1, "Java Programming", 3);
Course course2 = new Course(2, "Web Development", 4);
courseDAO.addCourse(course1);
courseDAO.addCourse(course2);
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getServletPath();
switch (action) {
case "/students":
listStudents(request, response);
break;
case "/courses":
listCourses(request, response);
break;
case "/enroll":
enrollCourse(request, response);
break;
case "/withdraw":
withdrawCourse(request, response);
break;
default:
response.sendRedirect("/");
break;
}
}
private void listStudents(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Student> students = studentDAO.getAllStudents();
request.setAttribute("students", students);
request.getRequestDispatcher("/students.jsp").forward(request, response);
}
private void listCourses(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Course> courses = courseDAO.getAllCourses();
request.setAttribute("courses", courses);
request.getRequestDispatcher("/courses.jsp").forward(request, response);
}
private void enrollCourse(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int studentId = Integer.parseInt(request.getParameter("studentId"));
int courseId = Integer.parseInt(request.getParameter("courseId"));
Student student = studentDAO.getStudentById(studentId);
Course course = courseDAO.getCourseById(courseId);
if (student != null && course != null) {
student.addCourse(course);
course.addStudent(student);
}
response.sendRedirect("/students");
}
private void withdrawCourse(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int studentId = Integer.parseInt(request.getParameter("studentId"));
int courseId = Integer.parseInt(request.getParameter("courseId"));
Student student = studentDAO.getStudentById(studentId);
Course course = courseDAO.getCourseById(courseId);
if (student != null && course != null) {
student.removeCourse(course);
course.removeStudent(student);
}
response.sendRedirect("/students");
}
}
```
students.jsp:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Student List</title>
</head>
<body>
<h1>Student List</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Courses</th>
</tr>
</thead>
<tbody>
<c:forEach items="${students}" var="student">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>
<ul>
<c:forEach items="${student.courses}" var="course">
<li>${course.name}</li>
</c:forEach>
</ul>
<form method="post" action="enroll">
<input type="hidden" name="studentId" value="${student.id}">
<select name="courseId">
<c:forEach items="${courses}" var="course">
<option value="${course.id}">${course.name}</option>
</c:forEach>
</select>
<button type="submit">Enroll</button>
</form>
<form method="post" action="withdraw">
<input type="hidden" name="studentId" value="${student.id}">
<select name="courseId">
<c:forEach items="${student.courses}" var="course">
<option value="${course.id}">${course.name}</option>
</c:forEach>
</select>
<button type="submit">Withdraw</button>
</form>
</td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
courses.jsp:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Course List</title>
</head>
<body>
<h1>Course List</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Credit</th>
<th>Students</th>
</tr>
</thead>
<tbody>
<c:forEach items="${courses}" var="course">
<tr>
<td>${course.id}</td>
<td>${course.name}</td>
<td>${course.credit}</td>
<td>
<ul>
<c:forEach items="${course.students}" var="student">
<li>${student.name}</li>
</c:forEach>
</ul>
</td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
以上代码只是一个简单的示例,仅供参考。实际开发中,还需要考虑数据持久化、安全性、性能等问题。
相关推荐
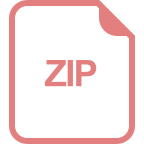
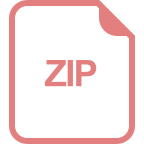
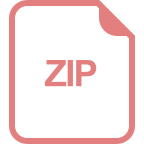














