根据前序扩展二叉树创建二叉链表二叉树,按中序遍历输出中序线索二叉树的ltag和rtag的值
时间: 2024-03-01 21:26:54 浏览: 63
首先,需要先将二叉链表转化为中序线索二叉树。中序线索二叉树的特点是,对于每一个节点,如果它有左子树,则它的ltag为0,指向它的左子树;如果它没有左子树,则它的ltag为1,指向它在中序遍历中的前驱节点。同样,如果它有右子树,则它的rtag为0,指向它的右子树;如果它没有右子树,则它的rtag为1,指向它在中序遍历中的后继节点。
下面是具体的实现过程:
1. 定义二叉树的数据结构
```python
class BiTreeNode:
def __init__(self, data=None):
self.data = data
self.left_child = None
self.right_child = None
self.ltag = 0
self.rtag = 0
```
2. 将二叉链表转化为中序线索二叉树
```python
def create_inorder_threaded_binary_tree(root):
pre = None
if root is not None:
create_inorder_threaded_binary_tree(root.left_child)
if root.left_child is None:
root.ltag = 1
root.left_child = pre
if pre is not None and pre.right_child is None:
pre.rtag = 1
pre.right_child = root
pre = root
create_inorder_threaded_binary_tree(root.right_child)
```
3. 输出中序线索二叉树的ltag和rtag的值
```python
def print_inorder_threaded_binary_tree(root):
p = root
while p is not None:
while p.ltag == 0:
p = p.left_child
print(p.data, p.ltag, p.rtag)
while p.rtag == 1 and p.right_child is not None:
p = p.right_child
print(p.data, p.ltag, p.rtag)
p = p.right_child
```
最后,调用以上三个函数即可完成该问题的求解。
阅读全文
相关推荐
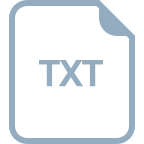
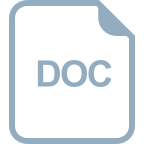
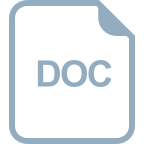

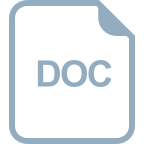
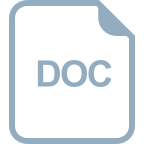
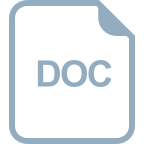
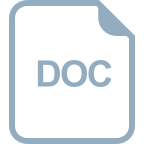
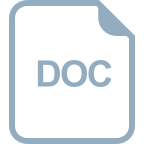
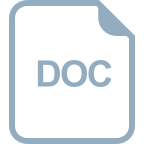
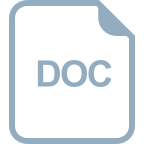



