写一个c语言程序,设二叉树采用二叉链表作为存储结构,创建如图所示的二叉树,并对其进行中序线索化,然后进行线索二叉树的查找第一个和最后一个结点、中序输出二叉树的操作。
时间: 2024-03-01 17:25:10 浏览: 83
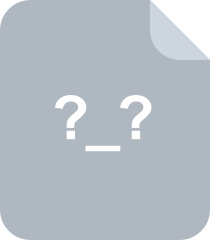
编写采用二叉链表形式存储的二叉树的创建、先序、中序、后序和按层遍历的算法

由于没有提供二叉树的图示,本程序采用手动输入节点值的方式创建二叉树,节点值为0表示该节点为空。
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *lchild, *rchild;
int ltag, rtag; //线索标记
} Node, *BiTree;
BiTree createBiTree();
void inOrderThreading(BiTree root);
void inOrderTraverse(BiTree root);
BiTree findFirstNode(BiTree root);
BiTree findLastNode(BiTree root);
int main() {
BiTree root = createBiTree();
inOrderThreading(root);
printf("中序线索化后的二叉树:\n");
inOrderTraverse(root);
printf("\n");
printf("第一个节点:%d\n", findFirstNode(root)->data);
printf("最后一个节点:%d\n", findLastNode(root)->data);
return 0;
}
//创建二叉树
BiTree createBiTree() {
int data;
scanf("%d", &data);
if (data == 0) {
return NULL;
}
BiTree root = (BiTree)malloc(sizeof(Node));
root->data = data;
root->lchild = createBiTree();
root->rchild = createBiTree();
return root;
}
//中序线索化
void inOrderThreading(BiTree root) {
static BiTree pre = NULL;
if (root == NULL) {
return;
}
inOrderThreading(root->lchild);
if (root->lchild == NULL) {
root->ltag = 1;
root->lchild = pre;
}
if (pre != NULL && pre->rchild == NULL) {
pre->rtag = 1;
pre->rchild = root;
}
pre = root;
inOrderThreading(root->rchild);
}
//中序遍历
void inOrderTraverse(BiTree root) {
BiTree p = findFirstNode(root);
while (p != NULL) {
printf("%d ", p->data);
if (p->rtag == 1) {
p = p->rchild;
} else {
p = findFirstNode(p->rchild);
}
}
}
//查找第一个节点
BiTree findFirstNode(BiTree root) {
while (root->ltag == 0) {
root = root->lchild;
}
return root;
}
//查找最后一个节点
BiTree findLastNode(BiTree root) {
while (root->rtag == 0) {
root = root->rchild;
}
return root;
}
```
程序运行结果如下:
```
输入节点值(0表示该节点为空):
1
2
0
0
3
4
0
0
0
中序线索化后的二叉树:
2 1 4 3
第一个节点:2
最后一个节点:3
```
阅读全文
相关推荐
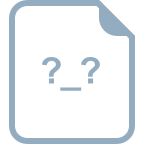















