if (! paths[i].empty()) { double cur_x = agvs[i].current_x; double cur_y = agvs[i].current_y; Node* next_node = paths[i][0]; float speed = agvs[i].getSpeed(); float distance = sqrt(pow(next_node->x - cur_x, 2) + pow(next_node->y - cur_y, 2)); int steps = ceil(distance / (speed * 0.01)); // 计算需要移动的步数 float power_consumption = distance / 20; for (int j = 1; j <= steps; j++) { float time = j * 100.0 / speed;// 每步所需时间 QTimer::singleShot(time, this, [&, i, next_node, cur_x, cur_y, power_consumption, j, steps]() { float ratio = static_cast<float>(j) / steps; double new_x = cur_x + ratio * (next_node->x - cur_x); double new_y = cur_y + ratio * (next_node->y - cur_y); agvs[i].setCurrentX(new_x); agvs[i].setCurrentY(new_y); std:: cout <<"AGV"<<"("<< new_x << "," << new_y << ")" << endl; // 更新电量 agvs[i].setpower(agvs[i].power_ - power_consumption / steps); this->update(); // 在窗口中重绘 }); } },添加代碼:在执行下一步移动前,先判断当前步骤的移动是否已经完成,如果未完成,就等待 QTimer 完成当前步骤的移动再进行下一步移动。
时间: 2024-01-27 13:05:23 浏览: 61
可以在每次启动 QTimer 时,将其返回的 QTimer 对象保存到一个数组中,然后在下一步移动前,判断前一步移动是否已完成,如果未完成,则等待 QTimer 完成当前步骤的移动再进行下一步移动。具体的代码如下:
```
QTimer* timer = nullptr;
QVector<QTimer*> timers;
if (! paths[i].empty()) {
double cur_x = agvs[i].current_x;
double cur_y = agvs[i].current_y;
Node* next_node = paths[i][0];
float speed = agvs[i].getSpeed();
float distance = sqrt(pow(next_node->x - cur_x, 2) + pow(next_node->y - cur_y, 2));
int steps = ceil(distance / (speed * 0.01)); // 计算需要移动的步数
float power_consumption = distance / 20;
for (int j = 1; j <= steps; j++) {
float time = j * 100.0 / speed;// 每步所需时间
timer = new QTimer(this);
timers.append(timer);
connect(timer, &QTimer::timeout, this, [=]() {
float ratio = static_cast<float>(j) / steps;
double new_x = cur_x + ratio * (next_node->x - cur_x);
double new_y = cur_y + ratio * (next_node->y - cur_y);
agvs[i].setCurrentX(new_x);
agvs[i].setCurrentY(new_y);
std::cout <<"AGV"<<"("<< new_x << "," << new_y << ")" << std::endl;
// 更新电量
agvs[i].setpower(agvs[i].power_ - power_consumption / steps);
this->update(); // 在窗口中重绘
});
if (!timers.isEmpty()) {
QTimer* preTimer = timers.last();
if (preTimer->isActive()) {
QTimer::singleShot(preTimer->remainingTime(), this, [=]() {
timer->start(time - preTimer->remainingTime());
});
} else {
timer->start(time);
}
} else {
timer->start(time);
}
}
}
```
这样,在每次启动 QTimer 时,就将其返回的 QTimer 对象保存到 `timers` 数组中,然后判断前一步移动是否已完成,如果未完成,则等待 QTimer 完成当前步骤的移动再进行下一步移动。这样可以避免 AGV 倒退的现象。
阅读全文
相关推荐
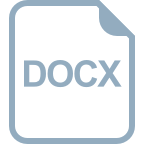
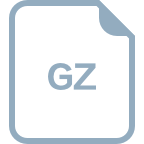
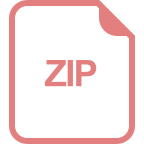





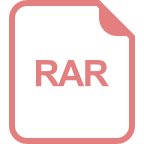
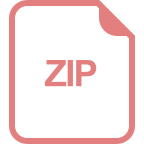