stm32f103 5*4键盘 代码
时间: 2023-11-04 16:04:41 浏览: 48
以下是一个使用STM32F103 MCU的5x4键盘代码示例,它使用了GPIO库和定时器库。
```c
#include "stm32f10x.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_tim.h"
#define ROWS 5
#define COLS 4
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_TypeDef* rowPorts[ROWS] = {GPIOA, GPIOA, GPIOA, GPIOA, GPIOA};
uint16_t rowPins[ROWS] = {GPIO_Pin_0, GPIO_Pin_1, GPIO_Pin_2, GPIO_Pin_3, GPIO_Pin_4};
GPIO_TypeDef* colPorts[COLS] = {GPIOB, GPIOB, GPIOB, GPIOB};
uint16_t colPins[COLS] = {GPIO_Pin_12, GPIO_Pin_13, GPIO_Pin_14, GPIO_Pin_15};
uint8_t keys[ROWS][COLS] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'},
{'X', 'X', 'X', 'X'}
};
void scanKeys() {
int i, j;
for(i = 0; i < ROWS; i++) {
GPIO_ResetBits(rowPorts[i], rowPins[i]);
for(j = 0; j < COLS; j++) {
if(GPIO_ReadInputDataBit(colPorts[j], colPins[j]) == RESET) {
// key pressed
printf("%c pressed\n", keys[i][j]);
while(GPIO_ReadInputDataBit(colPorts[j], colPins[j]) == RESET);
}
}
GPIO_SetBits(rowPorts[i], rowPins[i]);
}
}
void initGPIO() {
int i;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
for(i = 0; i < ROWS; i++) {
GPIO_InitStructure.GPIO_Pin = rowPins[i];
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(rowPorts[i], &GPIO_InitStructure);
GPIO_SetBits(rowPorts[i], rowPins[i]);
}
for(i = 0; i < COLS; i++) {
GPIO_InitStructure.GPIO_Pin = colPins[i];
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(colPorts[i], &GPIO_InitStructure);
}
}
void initTimer() {
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM4, ENABLE);
TIM_TimeBaseStructure.TIM_Period = 999;
TIM_TimeBaseStructure.TIM_Prescaler = 7199;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM4, &TIM_TimeBaseStructure);
TIM_Cmd(TIM4, ENABLE);
TIM_ITConfig(TIM4, TIM_IT_Update, ENABLE);
}
int main(void) {
initGPIO();
initTimer();
while(1) {
}
}
void TIM4_IRQHandler(void) {
if(TIM_GetITStatus(TIM4, TIM_IT_Update) != RESET) {
scanKeys();
TIM_ClearITPendingBit(TIM4, TIM_IT_Update);
}
}
```
这个简单的示例代码可用于5x4矩阵键盘扫描。它使用了定时器中断来周期性地扫描键盘,并在控制台上输出按下的键。你可以根据自己的需求修改keys数组以匹配你的键盘布局。
相关推荐
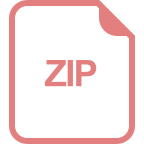
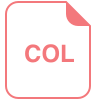
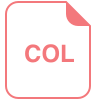
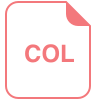
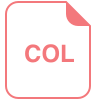
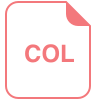









