使用python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集
时间: 2023-08-31 07:40:45 浏览: 213
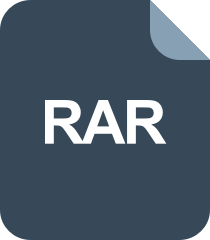
使用Python的石油资讯网络爬虫
### 回答1:
首先,你需要安装Python库'beautifulsoup4'和'requests',如果没有安装过,你可以在终端中使用'pip install beautifulsoup4'和'pip install requests'来安装。
然后你可以使用以下代码来爬取这个数据集:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection"
page = requests.get(url)
soup = BeautifulSoup(page.content, "html.parser")
download_url = soup.find_all("a", class_="gs-c-btn gs-c-btn--small gs-c-btn--primary gs-c-btn--inline")[0]["href"]
print(download_url)
```
这将会打印出数据集的下载链接。
之后,可以使用`wget`或者`urllib`来下载这个数据集。
```python
import wget
wget.download(download_url)
```
或者
```python
import urllib.request
urllib.request.urlretrieve(download_url, "data.zip")
```
### 回答2:
要使用Python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集,可以按照以下步骤进行:
1. 导入必要的库,例如`requests`和`BeautifulSoup`,确保你已经安装了它们。
2. 使用`requests`库发送GET请求获取网页的内容,可以使用以下代码:
```python
import requests
url = "https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection"
response = requests.get(url)
```
3. 使用`BeautifulSoup`库对获取的网页内容进行解析,这样我们可以提取出需要的数据。可以使用以下代码:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
```
4. 查找数据集下载链接的HTML元素,通常可以通过查看网页源代码确定。在这个例子中,下载链接位于一个`a`标签内,它的`href`属性指向数据集文件的URL。
5. 使用`find`或`find_all`方法从解析得到的`soup`对象中提取出下载链接。代码如下:
```python
download_link = soup.find('a', class_='dataset-download__button').get('href')
```
6. 可以使用`wget`库或`requests`库中的`get`方法来下载数据集文件。如果选择使用`wget`库,可以使用以下代码:
```python
import wget
file_path = "path/to/save/file" # 将文件保存到本地的路径
wget.download(download_link, file_path)
```
如果选择使用`requests`库,可以使用以下代码:
```python
with open(file_path, "wb") as file:
file.write(requests.get(download_link).content)
```
7. 现在,你已经成功地使用Python爬取了https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集,并将其保存到了本地。
请注意,在爬取任何网站时,请遵守网站的使用条款和条件,并确保你有合法的许可使用该数据集。
### 回答3:
使用Python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集可以执行以下步骤:
1. 安装所需的库:首先,需要安装requests和beautifulsoup库。可以使用pip install requests和pip install beautifulsoup进行安装。
2. 发送HTTP请求:使用requests库发送HTTP GET请求以获取网页的内容。可以使用以下代码行来实现:
```
import requests
url = "https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection"
response = requests.get(url)
```
3. 解析网页内容:使用beautifulsoup库解析网页源代码,以从中提取所需的数据集URL。可以使用以下代码行来实现:
```
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
dataset_url = soup.find("a", text="Download").get('href')
```
4. 下载数据集:使用requests库的get方法下载数据集。可以使用以下代码行来实现:
```
dataset_response = requests.get(dataset_url)
with open('oil_spill_dataset.zip', 'wb') as dataset_file:
dataset_file.write(dataset_response.content)
```
这段代码将数据集保存为名为"oil_spill_dataset.zip"的文件。
请注意,这只是基本的概述,您可能需要根据您的具体需求和数据集的结构进行调整和改进。同时,还需要处理相关的异常情况,例如请求错误或网页无法解析等问题。
阅读全文
相关推荐
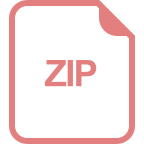











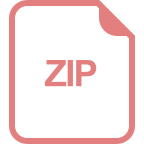
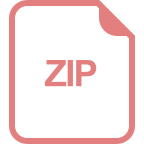