使用python爬取下面网址的https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection的数据集
时间: 2023-02-08 10:22:50 浏览: 270
可以使用 Python 库 "beautifulsoup4" 来爬取该网址的数据集。首先需要安装该库,可以在命令行中使用 "pip install beautifulsoup4" 安装。然后可以使用 "requests" 库发送网络请求来获取网页的 HTML 代码,再使用 "beautifulsoup4" 解析 HTML 代码并提取数据。
代码类似如下:
```
import requests
from bs4 import BeautifulSoup
url = "https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 使用beautifulsoup解析网页
# 代码继续提取数据
```
注意, kaggle 数据集下载需要登录, 如果需要爬取数据集, 建议通过 kaggle api 获取.
相关问题
使用python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集
好的,您可以按照以下步骤使用Python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集:
1. 导入要的库
```python
import requests
from bs4 import BeautifulSoup
import os
```
2. 发送请求并解析网页
```python
url = "https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
```
3. 查找下载链接
```python
download_link = soup.find("a", attrs={"id": "downloadButton"})['href']
```
4. 下载数据集
```python
response = requests.get(download_link, stream=True)
file_size = int(response.headers.get("Content-Length", 0))
filename = os.path.join(os.getcwd(), "oil_spill_detection.zip")
with open(filename, "wb") as f:
for data in response.iter_content(1024):
f.write(data)
```
这样,您就可以使用Python成功地爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集。
给出用python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection数据的代码
### 回答1:
下面是一个使用 Python 爬取 https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection 的代码示例:
```
import requests
url = 'https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection'
response = requests.get(url)
with open('oil-spill-detection.csv', 'wb') as f:
f.write(response.content)
```
这段代码使用了 Python 中 requests 库来发送 GET 请求,并将返回的数据保存在当前目录下的 oil-spill-detection.csv 文件中。
需要注意的是,如果这个数据集需要登录才能下载的话,需要额外的操作来登录才能爬取到数据。
### 回答2:
以下是使用Python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection数据的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 请求页面
url = 'https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection'
response = requests.get(url)
# 解析页面内容
soup = BeautifulSoup(response.text, 'html.parser')
# 查找下载链接
download_link = soup.find('div', class_='download-button-container') \
.find('a', class_='site-button')['href']
# 下载数据文件
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
data_response = requests.get(download_link, headers=headers)
# 保存数据文件
with open('oil_spill_detection.zip', 'wb') as file:
file.write(data_response.content)
print('数据爬取完成并保存成功!')
```
这段代码首先使用`requests`库发送HTTP请求,获取网页的HTML内容。然后,使用` BeautifulSoup`库解析HTML内容,查找下载链接的位置。接着,使用`requests`库再次发送HTTP请求,下载数据文件。最后,将下载的数据文件保存到本地。
相关推荐
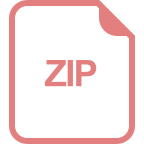
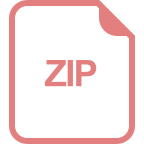
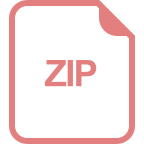









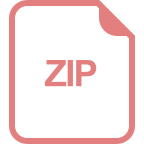
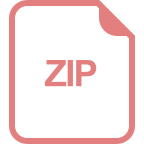
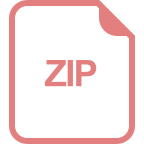