请帮编写代码,输出二叉树中从每个叶子结点到根结点的路径。
时间: 2023-12-29 14:05:47 浏览: 82
以下是 Python 代码实现,其中 `TreeNode` 表示二叉树节点,`findPaths` 函数接受一个二叉树根节点参数,返回一个包含各路径的列表:
```python
class TreeNode(object):
def __init__(self, x):
self.val = x
self.left = None
self.right = None
def findPaths(root):
res = []
path = []
dfs(root, res, path)
return res
def dfs(node, res, path):
if not node:
return
path.append(node.val)
if not node.left and not node.right:
res.append(path[::-1])
dfs(node.left, res, path)
dfs(node.right, res, path)
path.pop()
```
使用示例:
```python
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
root.right.left = TreeNode(6)
root.right.right = TreeNode(7)
paths = findPaths(root)
for path in paths:
print(path)
```
输出结果:
```
[4, 2, 1]
[5, 2, 1]
[6, 3, 1]
[7, 3, 1]
```
阅读全文
相关推荐
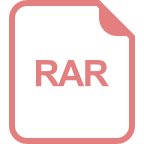
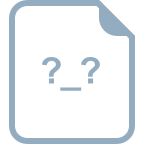















