汉诺塔,python代码
时间: 2023-08-31 07:40:46 浏览: 108
### 回答1:
汉诺塔是一个经典的递归问题。下面是一个用 Python 实现的汉诺塔解法的代码示例:
```
def hanoi(n, source, helper, target):
if n > 0:
# 将 n-1 个盘子从 source 移动到 helper
hanoi(n - 1, source, target, helper)
# 将第 n 个盘子从 source 移动到 target
if source:
target.append(source.pop())
# 将 n-1 个盘子从 helper 移动到 target
hanoi(n - 1, helper, source, target)
source = [4, 3, 2, 1]
target = []
helper = []
hanoi(len(source), source, helper, target)
print(source)
print(helper)
print(target)
```
这段代码中,我们定义了一个 `hanoi` 函数,用于递归地解决汉诺塔问题。它有三个参数:
- `n` 表示要移动的盘子数量。
- `source` 表示要移动的盘子所在的柱子。
- `helper` 和 `target` 分别表示辅助柱和目标柱。
在函数中,我们首先检查 `n` 的值是否大于 0,如果是,则执行三个步骤:
1. 将 `n-1` 个盘子从 `source` 移动到 `helper`。
2. 将第 `n` 个盘子从 `source` 移动到 `target`。
3. 将 `n-1` 个盘子从 `helper` 移动到 `target`。
每一步都调用自身,直到 `n` 等于 0 为止。
在函数外部,我们调用 `hanoi` 函数,并传入要移动的盘子数量,以及三个柱子的列表作为参
### 回答2:
汉诺塔是一个经典的数学问题,可以使用递归的方法来解决。
在Python中,可以使用以下代码来实现汉诺塔游戏:
```python
def hanoi(n, A, B, C):
if n == 1:
print("Move disk from", A, "to", C)
else:
hanoi(n-1, A, C, B)
print("Move disk from", A, "to", C)
hanoi(n-1, B, A, C)
n = 3 # 汉诺塔的层数
A = "A" # 起始柱子
B = "B" # 中间柱子
C = "C" # 目标柱子
hanoi(n, A, B, C)
```
上述代码中,`hanoi()`函数接受四个参数:`n`表示汉诺塔的层数,`A`、`B`和`C`分别表示起始柱子、中间柱子和目标柱子。
当层数`n`为1时,直接将叠在起始柱子上的唯一一个盘子移动到目标柱子上,并打印移动的过程。
当层数`n`大于1时,将`n-1`层的盘子从起始柱子经过目标柱子移动到中间柱子上,然后将剩下的一层最大盘子从起始柱子移动到目标柱子上,最后将中间柱子上的`n-1`层盘子移动到目标柱子上。在每个移动步骤中,都会打印出移动的过程。
通过运行上述代码,即可在控制台输出汉诺塔游戏的步骤信息。
阅读全文
相关推荐

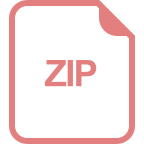









