不使用库的lin通讯C语言代码
时间: 2024-01-21 11:19:26 浏览: 21
Lin通讯是一种用于在汽车电子系统中进行通信的协议。以下是一个使用C语言实现Lin通讯的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#include <termios.h>
#define LIN_MSG_LEN 8
#define LIN_MSG_BREAK 0x00
#define LIN_MSG_SYNC 0x55
#define LIN_MSG_ID 0x01
#define LIN_MSG_P1 0x00
#define LIN_MSG_P2 0x00
#define LIN_MSG_P3 0x00
#define LIN_MSG_P4 0x00
#define LIN_MSG_CHECKSUM (LIN_MSG_ID + LIN_MSG_P1 + LIN_MSG_P2 + LIN_MSG_P3 + LIN_MSG_P4)
void set_interface_attribs(int fd, int speed) {
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(fd, &tty) != 0) {
printf("error %d from tcgetattr", errno);
exit(EXIT_FAILURE);
}
cfsetospeed(&tty, (speed_t)speed);
cfsetispeed(&tty, (speed_t)speed);
tty.c_cflag |= (CLOCAL | CREAD); /* ignore modem controls */
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8; /* 8-bit characters */
tty.c_cflag &= ~PARENB; /* no parity bit */
tty.c_cflag &= ~CSTOPB; /* only need 1 stop bit */
tty.c_cflag &= ~CRTSCTS; /* no hardware flowcontrol */
/* setup for non-canonical mode */
tty.c_iflag &= ~(IGNBRK | BRKINT | PARMRK | ISTRIP | INLCR | IGNCR | ICRNL | IXON);
tty.c_lflag &= ~(ECHO | ECHONL | ICANON | ISIG | IEXTEN);
tty.c_oflag &= ~OPOST;
/* fetch bytes as they become available */
tty.c_cc[VMIN] = 1;
tty.c_cc[VTIME] = 1;
if (tcsetattr(fd, TCSANOW, &tty) != 0) {
printf("error %d from tcsetattr", errno);
exit(EXIT_FAILURE);
}
}
int main() {
char *portname = "/dev/ttyUSB0";
int fd = open(portname, O_RDWR | O_NOCTTY | O_SYNC);
if (fd < 0) {
printf("error %d opening %s: %s", errno, portname, strerror(errno));
exit(EXIT_FAILURE);
}
set_interface_attribs(fd, B9600);
unsigned char tx_buffer[LIN_MSG_LEN];
tx_buffer[0] = LIN_MSG_BREAK;
tx_buffer[1] = LIN_MSG_SYNC;
tx_buffer[2] = LIN_MSG_ID;
tx_buffer[3] = LIN_MSG_P1;
tx_buffer[4] = LIN_MSG_P2;
tx_buffer[5] = LIN_MSG_P3;
tx_buffer[6] = LIN_MSG_P4;
tx_buffer[7] = LIN_MSG_CHECKSUM;
ssize_t num_bytes_written = write(fd, tx_buffer, LIN_MSG_LEN);
if (num_bytes_written != LIN_MSG_LEN) {
printf("error %d from write: %s", errno, strerror(errno));
exit(EXIT_FAILURE);
}
close(fd);
return EXIT_SUCCESS;
}
```
此代码使用Linux系统的串行通信API进行通信。要使用此代码,您需要将端口名称(portname)替换为您的系统中的实际串行端口名称,并根据需要更改波特率。此示例代码发送一个长度为8字节的Lin消息,其中包含一个同步字节、一个消息ID和四个字节的数据。在发送数据之前,该代码通过写入BREAK和SYNC字节来在总线上发送同步信号。
相关推荐
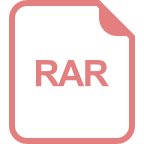
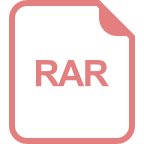














