请用c语言写一个二叉排序树,并排序数字1到20
时间: 2023-06-01 20:04:22 浏览: 92
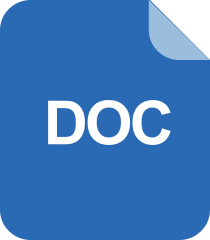
二叉排序树 c 版本
```
#include <stdio.h>
#include <stdlib.h>
// 定义二叉排序树结点结构体
typedef struct BSTNode {
int data; // 数据域
struct BSTNode *lchild; // 左孩子指针
struct BSTNode *rchild; // 右孩子指针
} BSTNode, *BSTree;
// 初始化二叉排序树
void initBSTree(BSTree *T) {
*T = NULL;
}
// 插入结点到二叉排序树中
int insertBSTree(BSTree *T, int key) {
// 如果树为空,创建新结点,插入到根节点
if (*T == NULL) {
*T = (BSTNode *)malloc(sizeof(BSTNode));
(*T)->data = key;
(*T)->lchild = NULL;
(*T)->rchild = NULL;
return 1;
}
// 如果树不为空
// 如果插入的值已经存在于树中,返回0
if (key == (*T)->data) {
return 0;
}
// 如果插入的值小于根节点,将其插入到左子树中
else if (key < (*T)->data) {
return insertBSTree(&((*T)->lchild), key);
}
// 如果插入的值大于根节点,将其插入到右子树中
else {
return insertBSTree(&((*T)->rchild), key);
}
}
// 中序遍历二叉排序树
void inOrderBSTree(BSTree T) {
if (T != NULL) {
inOrderBSTree(T->lchild);
printf("%d ", T->data);
inOrderBSTree(T->rchild);
}
}
int main() {
BSTree T;
int i, a[20] = {5, 10, 3, 8, 7, 15, 6, 12, 9, 1, 14, 2, 17, 4, 20, 18, 13, 16, 11, 19};
initBSTree(&T);
// 将数字插入到二叉排序树中
for (i = 0; i < 20; i++) {
insertBSTree(&T, a[i]);
}
// 中序遍历二叉排序树,输出排序结果
inOrderBSTree(T);
return 0;
}
```
阅读全文
相关推荐
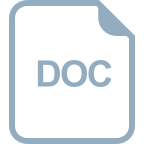
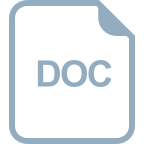


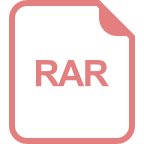










