import torch a = torch.tensor([2, 3, 4]) b = torch.tensor([2, 3, 4]) c =torch.add(a,b) print(c)
时间: 2024-05-20 14:16:57 浏览: 59
The output of this code will be:
tensor([4, 6, 8])
This is because we are adding two tensors a and b element-wise using the torch.add() function, and the resulting tensor c contains the sum of each corresponding element in a and b. In other words, c[i] = a[i] + b[i] for each i in the range of the tensor dimensions.
相关问题
import torch x = torch.tensor([]) y = torch.tensor([1, 2, 3]) z = torch.cat((x, y), dim = 1)
这段代码会报错,因为在对 x 进行拼接时,x 是一个空的张量,没有指定维度。正确的写法应该是:
```
import torch
x = torch.tensor([])
y = torch.tensor([1, 2, 3])
z = torch.cat((x.view(1,-1), y.view(1,-1)), dim = 1)
```
这里使用了 `view` 方法将 x 和 y 的维度都转换成 `(1, -1)`,然后在第二维上进行拼接。
x=torch.tensor(1.) w1=torch.tensor(2.,requires_grad=True) b1=torch.tensor(1.) w2=torch.tensor(2.,requires_grad=True) b2=torch.tensor(1.) y1=x*w1+b1 y2=y1*w2+b2 dy2_dy1=autograd.grad(y2,[y1],retain_graph=True)[0] dy1_dw1=autograd.grad(y1,[w1],retain_graph=True)[0] dy2_dw1=autograd.grad(y2,[w1],retain_graph=True)[0] dy2_dy1*dy1_dw1 dy2_dw1
这段代码中有一个小错误,第四行的y1计算时少写了一个乘号。正确的代码如下:
```
x=torch.tensor(1.)
w1=torch.tensor(2.,requires_grad=True)
b1=torch.tensor(1.)
w2=torch.tensor(2.,requires_grad=True)
b2=torch.tensor(1.)
y1=x*w1+b1
y2=y1*w2+b2
dy2_dy1=torch.autograd.grad(y2,[y1],retain_graph=True)[0]
dy1_dw1=torch.autograd.grad(y1,[w1],retain_graph=True)[0]
dy2_dw1=torch.autograd.grad(y2,[w1],retain_graph=True)[0]
dy2_dy1*dy1_dw1
dy2_dw1
```
接下来回答你的问题:
这段代码中,dy2_dy1是y2对y1的导数,dy1_dw1是y1对w1的导数,dy2_dw1是y2对w1的导数。因此,dy2_dy1*dy1_dw1就是y2对w1的二阶导数,而dy2_dw1就是y2对w1的一阶导数。
阅读全文
相关推荐
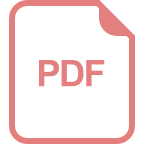















