用C语言写一份程序,从0~100均匀分布中进行抽样,然后获取其均值,并输出均值其在0~100上的概率分布图
时间: 2024-10-04 19:04:38 浏览: 65
在C语言中编写一个程序来实现这个功能需要几个步骤,包括生成随机数、计算平均值以及绘制概率分布图。由于C语言本身并不支持直接绘制图形,我们可以借助外部库如`gnuplot`来辅助绘制。这里是一个简单的示例,首先通过`<stdlib.h>`和`<time.h>`库生成随机数,计算平均值,然后通过命令行调用`gnuplot`进行可视化。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_VALUE 100
#define SAMPLE_SIZE 1000
void generate_random_samples(int samples[], int max_value) {
srand(time(0));
for (int i = 0; i < SAMPLE_SIZE; i++) {
samples[i] = rand() % max_value;
}
}
double calculate_average(int samples[]) {
double sum = 0;
for (int i = 0; i < SAMPLE_SIZE; i++) {
sum += samples[i];
}
return sum / SAMPLE_SIZE;
}
void plot_distribution(int samples[], int max_value) {
FILE *gnuplot_file = popen("gnuplot -persist", "w");
fprintf(gnuplot_file, "set terminal png\n");
fprintf(gnuplot_file, "set output 'probability_distribution.png'\n");
fprintf(gnuplot_file, "binwidth = 1\n");
fprintf(gnuplot_file, "plot '-' using 2:xtic(1) with boxes title 'Probability Distribution',\n");
for (int i = 0; i <= max_value; i++) {
int count = 0;
for (int j = 0; j < SAMPLE_SIZE; j++) {
if (samples[j] >= i && samples[j] < i + binwidth) {
count++;
}
}
fprintf(gnuplot_file, "%d * %.1f w l t \"Value=%d\"\n", count, count / SAMPLE_SIZE, i);
}
fprintf(gnuplot_file, "e\n");
pclose(gnuplot_file);
}
int main() {
int samples[SAMPLE_SIZE];
generate_random_samples(samples, MAX_VALUE);
double average = calculate_average(samples);
printf("Average value: %.2lf\n", average);
plot_distribution(samples, MAX_VALUE);
return 0;
}
```
当你运行此程序时,它会生成一个名为`probability_distribution.png`的图像文件,显示0到100之间随机样本的概率分布。注意,你需要在你的系统上安装`gnuplot`才能运行此代码。如果你的系统上没有,你可以从`gnuplot.org`下载并安装。
阅读全文
相关推荐
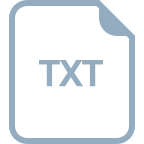
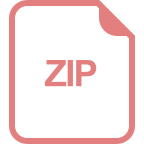
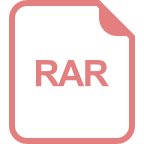







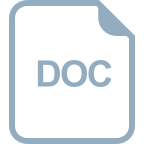
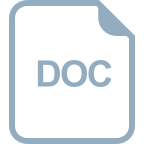
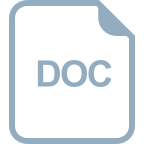
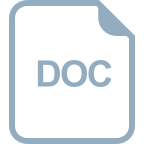
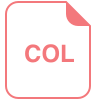
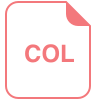
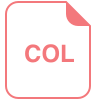


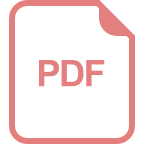