class Net(nn.Module): def __init__(self): super(Net,self).__init__() self.fc1=nn.Linear(3,10) self.fc2=nn.Linear(10,1) def forward(self, x): x=self.fc1(x) x=torch.relu(x) x=self.fc2(x) return x net = Net()逐行解释
时间: 2023-11-21 13:06:21 浏览: 56
逐行解释以下代码:
```python
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(3, 10)
self.fc2 = nn.Linear(10, 1)
def forward(self, x):
x = self.fc1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
net = Net()
```
- `class Net(nn.Module):`:定义一个名为 `Net` 的类,继承自 `nn.Module` 类,即 `Net` 类是一个 PyTorch 神经网络模型。
- `def __init__(self):`:定义 `Net` 类的初始化函数。
- `super(Net, self).__init__()`:调用父类的初始化函数,即 `nn.Module` 类的初始化函数。
- `self.fc1 = nn.Linear(3, 10)`:定义一个全连接层 `fc1`,将输入的特征向量的维度从 `3` 转换为 `10`,其中 `nn.Linear` 是 PyTorch 中的一个线性变换(全连接层)模块。
- `self.fc2 = nn.Linear(10, 1)`:定义一个全连接层 `fc2`,将输入的特征向量的维度从 `10` 转换为 `1`。
- `def forward(self, x):`:定义 `Net` 类的前向传播函数,即对输入 `x` 进行一次前向传播计算。
- `x = self.fc1(x)`:将输入 `x` 输入到全连接层 `fc1` 中进行线性变换,得到一个新的张量 `x`。
- `x = torch.relu(x)`:将张量 `x` 输入到 ReLU 激活函数中,得到一个新的张量 `x`,ReLU 函数的作用是将所有小于零的值都置零,保留所有大于等于零的值。
- `x = self.fc2(x)`:将张量 `x` 输入到全连接层 `fc2` 中进行线性变换,得到最终的输出张量 `x`。
- `return x`:返回输出张量 `x`。
- `net = Net()`:创建一个 `Net` 类的实例,即创建了一个 PyTorch 神经网络模型。
相关推荐
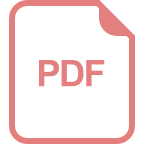
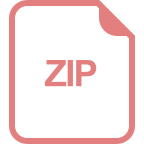
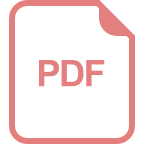















