能帮我写一个elgamal算法用C++
时间: 2024-05-07 21:21:40 浏览: 105
当然可以,以下是一个简单的 ElGamal 加密/解密算法的 C++ 实现:
```cpp
#include <iostream>
#include <random>
#include <cmath>
using namespace std;
// 定义一个模数 p 和一个原根 g
const int p = 23;
const int g = 5;
// 生成一个随机数
int generateRandomNumber(int min, int max) {
random_device rd;
mt19937 gen(rd());
uniform_int_distribution<> distr(min, max);
return distr(gen);
}
// 计算模数幂
int power(int a, int b, int m) {
int res = 1;
while (b > 0) {
if (b & 1) res = (res * a) % m;
a = (a * a) % m;
b >>= 1;
}
return res;
}
// 计算模数逆元
int modInverse(int a, int m) {
int m0 = m, t, q;
int x0 = 0, x1 = 1;
if (m == 1) return 0;
while (a > 1) {
q = a / m;
t = m;
m = a % m, a = t;
t = x0;
x0 = x1 - q * x0;
x1 = t;
}
if (x1 < 0) x1 += m0;
return x1;
}
// 加密函数
void encrypt(int& c1, int& c2, int m, int y) {
int k = generateRandomNumber(1, p - 2);
c1 = power(g, k, p);
int temp = power(y, k, p);
c2 = (m * temp) % p;
}
// 解密函数
int decrypt(int c1, int c2, int x) {
int temp = power(c1, x, p);
int inv = modInverse(temp, p);
return (c2 * inv) % p;
}
int main() {
int x = generateRandomNumber(1, p - 2);
int y = power(g, x, p);
cout << "公钥为 (" << p << ", " << g << ", " << y << ")" << endl;
cout << "私钥为 " << x << endl;
int m;
cout << "请输入要加密的明文: ";
cin >> m;
int c1, c2;
encrypt(c1, c2, m, y);
cout << "密文为 (" << c1 << ", " << c2 << ")" << endl;
int decrypted = decrypt(c1, c2, x);
cout << "解密后的明文为 " << decrypted << endl;
return 0;
}
```
在此代码中,我们定义了一个模数 `p` 和一个原根 `g`。我们随机生成一个私钥 `x`,并计算公钥 `y`。然后,我们输入要加密的明文,并使用 `encrypt` 函数进行加密。最后,我们使用 `decrypt` 函数对密文进行解密,得到原始的明文。
注意,此代码中使用的是非常小的模数和密钥,仅用于演示目的。在实际应用中,应该使用更大的模数和密钥。
阅读全文
相关推荐






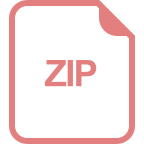
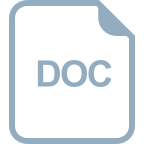







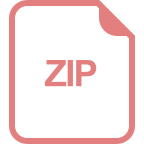
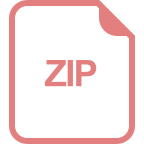