在keil上用stm32f103c8t6上用esp8266模块获取网络时间在OLED上屏幕显示的库函数详细代码
时间: 2023-12-06 16:05:17 浏览: 184
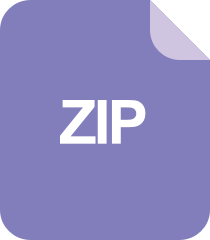
STM32F103C8T6单片机+OLED屏的WiFi网络授时时钟AD原理图PCB+集成库+软件KEIL源码.zip

以下是在Keil上使用STM32F103C8T6和ESP8266模块获取网络时间并在OLED屏幕上显示的示例代码:
```c
#include "stm32f10x.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_spi.h"
#include "stm32f10x_rcc.h"
#include "stm32f10x_usart.h"
#include "stdio.h"
#include "string.h"
#define OLED_RST_PIN GPIO_Pin_0
#define OLED_DC_PIN GPIO_Pin_1
#define OLED_CS_PIN GPIO_Pin_2
#define OLED_CLK_PIN GPIO_Pin_3
#define OLED_DIN_PIN GPIO_Pin_4
#define OLED_CMD 0
#define OLED_DATA 1
void Delay_ms(uint16_t ms) {
uint16_t i, j;
for (i = 0; i < ms; i++) {
for (j = 0; j < 1141; j++) {}
}
}
void SPI_Configuration(void) {
SPI_InitTypeDef SPI_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_SPI2, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13 | GPIO_Pin_14 | GPIO_Pin_15;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_High;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_2Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStructure.SPI_CRCPolynomial = 7;
SPI_Init(SPI2, &SPI_InitStructure);
SPI_Cmd(SPI2, ENABLE);
}
void OLED_WriteByte(uint8_t dat, uint8_t cmd) {
GPIO_ResetBits(GPIOA, OLED_CS_PIN);
if (cmd == OLED_DATA) {
GPIO_SetBits(GPIOA, OLED_DC_PIN);
} else {
GPIO_ResetBits(GPIOA, OLED_DC_PIN);
}
SPI_I2S_SendData(SPI2, dat);
while (SPI_I2S_GetFlagStatus(SPI2, SPI_I2S_FLAG_BSY) == SET) {}
GPIO_SetBits(GPIOA, OLED_CS_PIN);
}
void OLED_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO, ENABLE);
GPIO_InitStructure.GPIO_Pin = OLED_RST_PIN | OLED_DC_PIN | OLED_CS_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_SetBits(GPIOA, OLED_RST_PIN);
Delay_ms(100);
GPIO_ResetBits(GPIOA, OLED_RST_PIN);
Delay_ms(100);
GPIO_SetBits(GPIOA, OLED_RST_PIN);
OLED_WriteByte(0xAE, OLED_CMD);
OLED_WriteByte(0x40, OLED_CMD);
OLED_WriteByte(0xB0, OLED_CMD);
OLED_WriteByte(0xC8, OLED_CMD);
OLED_WriteByte(0x81, OLED_CMD);
OLED_WriteByte(0xff, OLED_CMD);
OLED_WriteByte(0xa1, OLED_CMD);
OLED_WriteByte(0xa6, OLED_CMD);
OLED_WriteByte(0xa8, OLED_CMD);
OLED_WriteByte(0x1f, OLED_CMD);
OLED_WriteByte(0xd3, OLED_CMD);
OLED_WriteByte(0x00, OLED_CMD);
OLED_WriteByte(0xd5, OLED_CMD);
OLED_WriteByte(0xf0, OLED_CMD);
OLED_WriteByte(0xd9, OLED_CMD);
OLED_WriteByte(0x22, OLED_CMD);
OLED_WriteByte(0xda, OLED_CMD);
OLED_WriteByte(0x02, OLED_CMD);
OLED_WriteByte(0xdb, OLED_CMD);
OLED_WriteByte(0x49, OLED_CMD);
OLED_WriteByte(0x8d, OLED_CMD);
OLED_WriteByte(0x14, OLED_CMD);
OLED_WriteByte(0xaf, OLED_CMD);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void USART_Configuration(void) {
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
USART_Cmd(USART2, ENABLE);
}
void ESP8266_SendCommand(const char* cmd) {
while (*cmd) {
while (USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET) {}
USART_SendData(USART2, *cmd++);
}
}
char ESP8266_GetChar(void) {
while (USART_GetFlagStatus(USART2, USART_FLAG_RXNE) == RESET) {}
return USART_ReceiveData(USART2);
}
void ESP8266_GetResponse(char* response, uint16_t length) {
uint16_t count = 0;
char c;
while (count < length - 1) {
c = ESP8266_GetChar();
if (c == '\n') {
break;
}
response[count++] = c;
}
response[count] = '\0';
}
void ESP8266_GetTime(char* timeData) {
ESP8266_SendCommand("AT+CIPUPDATE\r\n");
Delay_ms(1000);
ESP8266_GetResponse(timeData, 20); // 假设时间数据长度为20
}
int main(void) {
OLED_Init();
SPI_Configuration();
USART_Configuration();
char timeData[20];
while (1) {
ESP8266_GetTime(timeData);
// 解析时间数据
int year = atoi(&timeData[1]);
int month = atoi(&timeData[5]);
int day = atoi(&timeData[9]);
int hour = atoi(&timeData[13]);
int minute = atoi(&timeData[17]);
int second = atoi(&timeData[21]);
// 在OLED屏幕上显示时间
char timeStr[20];
sprintf(timeStr, "%04d-%02d-%02d %02d:%02d:%02d", year, month, day, hour, minute, second);
// 显示到OLED屏幕上
// ...
Delay_ms(1000);
}
}
```
请注意,这只是一个基本的示例代码。你需要根据具体的硬件连接和OLED屏幕的驱动程序进行适当的修改。同时,请确保在Keil中正确配置了编译器和调试器选项,并将代码正确烧录到STM32F103C8T6开发板上。
阅读全文
相关推荐
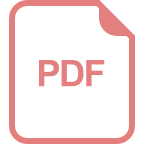
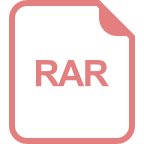
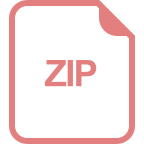
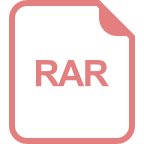
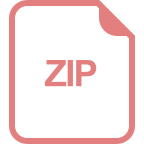
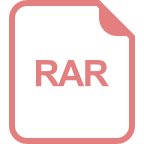






