Linux的RS485-RTU通讯编程
时间: 2024-02-01 12:36:05 浏览: 22
RS485是一种串行通信接口,可用于在长距离和噪声环境下进行数据通信。在Linux中,RS485-RTU通讯编程可以通过串口通信来实现。
以下是一个使用C语言编写的简单的RS485-RTU通讯程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
#define BAUDRATE B9600 // 通讯波特率
#define DEVICE "/dev/ttyS1"// 串口设备路径
#define TIMEOUT 10 // 超时时间(秒)
int fd;
int open_port(void) {
struct termios options;
fd = open(DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open_port: Unable to open serial port");
return -1;
}
fcntl(fd, F_SETFL, 0);
tcgetattr(fd, &options);
cfsetispeed(&options, BAUDRATE);
cfsetospeed(&options, BAUDRATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_oflag &= ~OPOST;
options.c_cc[VTIME] = TIMEOUT;
options.c_cc[VMIN] = 0;
tcsetattr(fd, TCSANOW, &options);
return fd;
}
int send_data(unsigned char *data, int length) {
int i;
int count;
count = write(fd, data, length);
if (count < 0) {
perror("send_data: Unable to send data");
return -1;
}
printf("send_data: ");
for (i = 0; i < count; i++) {
printf("%02x ", data[i]);
}
printf("\n");
return count;
}
int receive_data(unsigned char *data, int length) {
int i;
int count;
count = read(fd, data, length);
if (count < 0) {
perror("receive_data: Unable to receive data");
return -1;
}
printf("receive_data: ");
for (i = 0; i < count; i++) {
printf("%02x ", data[i]);
}
printf("\n");
return count;
}
int main() {
unsigned char send_buffer[] = {0x01, 0x03, 0x00, 0x00, 0x00, 0x01, 0x84, 0x0a};
unsigned char receive_buffer[255];
int count;
open_port();
send_data(send_buffer, sizeof(send_buffer));
count = receive_data(receive_buffer, sizeof(receive_buffer));
close(fd);
return 0;
}
```
在这个例子中,open_port()函数用于打开串口设备并配置通讯参数。send_data()函数用于发送数据,receive_data()函数用于接收数据。
该程序的示例中,发送了一个MODBUS RTU请求,然后等待接收一个响应。在实际应用中,可以根据需要修改发送和接收的数据,并根据协议规范进行处理。
相关推荐
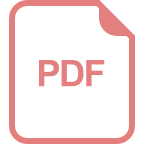














