用java代码写一个华容道
时间: 2023-05-15 07:07:09 浏览: 243
华容道是一种益智游戏,可以用Java代码来实现。以下是一个简单的华容道实现:
```java
import java.util.*;
public class HuaRongDao {
private int[][] board; // 棋盘
private int emptyRow; // 空格所在行
private int emptyCol; // 空格所在列
public HuaRongDao(int[][] board) {
this.board = board;
// 找到空格的位置
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[0].length; j++) {
if (board[i][j] == 0) {
emptyRow = i;
emptyCol = j;
break;
}
}
}
}
// 移动棋子
public boolean move(int row, int col) {
// 判断是否越界
if (row < 0 || row >= board.length || col < 0 || col >= board[0].length) {
return false;
}
// 判断是否与空格相邻
if (Math.abs(row - emptyRow) + Math.abs(col - emptyCol) != 1) {
return false;
}
// 交换棋子和空格的位置
int temp = board[row][col];
board[row][col] = board[emptyRow][emptyCol];
board[emptyRow][emptyCol] = temp;
emptyRow = row;
emptyCol = col;
return true;
}
// 判断是否完成
public boolean isFinished() {
int num = 1;
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[0].length; j++) {
if (board[i][j] != num && !(i == board.length - 1 && j == board[0].length - 1)) {
return false;
}
num++;
}
}
return true;
}
// 打印棋盘
public void printBoard() {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[0].length; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
int[][] board = {{1, 2, 3}, {4, 5, 6}, {7, 8, 0}}; // 初始棋盘
HuaRongDao game = new HuaRongDao(board);
Scanner scanner = new Scanner(System.in);
while (!game.isFinished()) {
game.printBoard();
System.out.print("请输入要移动的棋子的行和列(用空格隔开): ");
int row = scanner.nextInt();
int col = scanner.nextInt();
if (!game.move(row, col)) {
System.out.println("移动失败,请重新输入!");
}
}
System.out.println("恭喜你完成了华容道!");
}
}
```
这个程序实现了一个简单的华容道游戏,可以通过输入要移动的棋子的行和列来移动棋子,直到完成游戏。
相关推荐
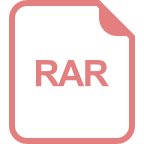
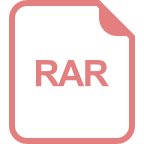














