基于tensorflow2.5,使用winograd算法编写一个可以直接插入神经网络使用的优化的深度可分离卷积模块并进行代码注释,解释其总体结构然后示例如何在神经网络中使用
时间: 2024-05-14 22:11:52 浏览: 85
首先,介绍一下深度可分离卷积(Depthwise Separable Convolution)。它是一种轻量级卷积操作,可以在减少参数数量的同时保持模型的准确性。它由两个步骤组成:深度卷积和逐点卷积。深度卷积是在每个输入通道上分别执行卷积,而逐点卷积是在所有通道之间执行卷积。这两个步骤的组合可以大大减少计算量,同时保持准确性。
Winograd算法是一种优化卷积计算的方法,可以减少计算量和存储空间,并提高计算速度。它使用小的卷积核,通过预计算转换矩阵来减少计算量。
下面是一个基于tensorflow2.5和Winograd算法的深度可分离卷积模块的代码:
```python
import tensorflow as tf
class WinogradDepthwiseSeparableConv2D(tf.keras.layers.Layer):
def __init__(self, filters, kernel_size, strides=(1, 1), padding='same', activation=None):
super(WinogradDepthwiseSeparableConv2D, self).__init__()
self.filters = filters
self.kernel_size = kernel_size
self.strides = strides
self.padding = padding
self.activation = tf.keras.activations.get(activation)
self.depthwise_conv = tf.keras.layers.DepthwiseConv2D(kernel_size=kernel_size, strides=strides, padding=padding, use_bias=False)
self.pointwise_conv = tf.keras.layers.Conv2D(filters=filters, kernel_size=(1, 1), strides=(1, 1), padding='valid', use_bias=True)
# Winograd算法需要的中间矩阵
self.winograd_coefficients = tf.constant([[1, 0, 0], [1/2, 1/2, 1/2], [1/2, -1/2, 1/2], [0, 0, 1], [0, 1, -1], [0, -1, -1]], dtype=tf.float32)
def call(self, inputs):
# 深度卷积
x = self.depthwise_conv(inputs)
# Winograd变换
x = tf.transpose(x, perm=[0, 3, 1, 2]) # 转置
x = tf.reshape(x, [-1, x.shape[2], x.shape[3]]) # 变形
x = tf.matmul(self.winograd_coefficients, x) # 矩阵乘法
x = tf.transpose(x, perm=[1, 2, 0]) # 转置
x = tf.reshape(x, [-1, x.shape[2], x.shape[0], 3]) # 变形
x = tf.transpose(x, perm=[0, 2, 1, 3]) # 转置
x = tf.reshape(x, [-1, x.shape[1], x.shape[2]*x.shape[3]]) # 变形
# 逐点卷积
x = self.pointwise_conv(x)
# 激活函数
if self.activation is not None:
x = self.activation(x)
return x
```
这个模块由两个卷积层组成:深度卷积和逐点卷积。在深度卷积之后,我们使用Winograd变换来减少计算量。变换的过程可以总结为以下步骤:
1. 转置输入张量的维度,使其变为(batch_size, input_height, input_width, input_channels)。
2. 将输入张量变形为(batch_size * input_height * input_width, input_channels)。
3. 将Winograd系数矩阵和输入张量相乘。
4. 将结果转置,并将其变形为(batch_size, output_height, output_width, 6)。
5. 转置输出张量的维度,使其变为(batch_size, 6, output_height, output_width)。
6. 将输出张量变形为(batch_size * output_height * output_width, 6)。
在逐点卷积之后,我们应用激活函数(如果有)并返回输出张量。
示例代码如下:
```python
import tensorflow as tf
inputs = tf.keras.Input(shape=(224, 224, 3))
x = WinogradDepthwiseSeparableConv2D(filters=32, kernel_size=(3, 3), strides=(2, 2))(inputs)
x = WinogradDepthwiseSeparableConv2D(filters=64, kernel_size=(3, 3), strides=(1, 1))(x)
x = WinogradDepthwiseSeparableConv2D(filters=128, kernel_size=(3, 3), strides=(2, 2))(x)
x = WinogradDepthwiseSeparableConv2D(filters=256, kernel_size=(3, 3), strides=(1, 1))(x)
x = WinogradDepthwiseSeparableConv2D(filters=512, kernel_size=(3, 3), strides=(2, 2))(x)
x = WinogradDepthwiseSeparableConv2D(filters=1024, kernel_size=(3, 3), strides=(1, 1))(x)
outputs = tf.keras.layers.GlobalAveragePooling2D()(x)
model = tf.keras.Model(inputs=inputs, outputs=outputs)
```
在这个示例中,我们创建了一个具有6个Winograd深度可分离卷积层的模型,每个卷积层的输出通道数从32到1024不等。最终,我们应用全局平均池化来生成模型的输出张量。
阅读全文
相关推荐
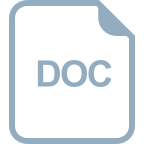
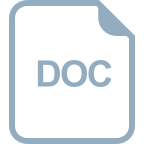
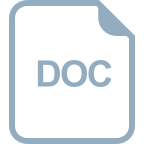









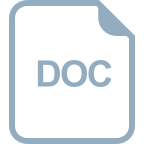
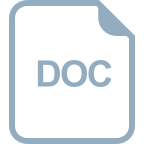
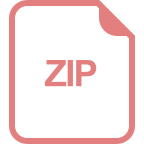
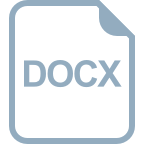