TypeError: can only concatenate tuple (not "str") to tuple
时间: 2023-07-12 15:36:55 浏览: 99
这个错误通常发生在试图将字符串和元组进行拼接时。例如:
```python
a = "hello"
b = ("world",)
c = a + b # TypeError: can only concatenate tuple (not "str") to tuple
```
要解决这个问题,需要将元组转换为字符串,或者将字符串转换为元组。具体的解决方法取决于你的代码和逻辑。
如果你想将元组转换为字符串,你可以使用 `join()` 方法。例如:
```python
a = "hello"
b = ("world",)
c = a + " " + "".join(b)
print(c) # 输出:hello world
```
如果你想将字符串转换为元组,你可以使用 `tuple()` 方法。例如:
```python
a = "hello"
b = tuple(a)
print(b) # 输出:('h', 'e', 'l', 'l', 'o')
```
希望这些解决方法能够帮助你解决问题!
相关问题
TypeError: can only concatenate str (not "tuple") to str
这个错误通常是由于您试图将字符串和元组连接起来而导致的。Python中的字符串和元组是两种不同类型的数据,不能直接连接起来。如果您想将字符串和元组连接起来,可以使用字符串格式化操作或将元组转换为字符串后再连接它们。下面是两种解决方法:
使用字符串格式化操作:
```python
my_tuple = (1, 2, 3)
my_string = "The tuple values are: %s" % str(my_tuple)
```
将元组转换为字符串后再连接它们:
```python
my_tuple = (1, 2, 3)
my_string = "The tuple values are: " + str(my_tuple)
```
在这两种情况下,元组都被转换为字符串,然后与另一个字符串连接起来,避免了TypeError。
TypeError: can only concatenate str (not "tuple") to str报错了
这个错误通常是由于字符串和元组不能直接相加导致的。根据错误提示,你可能在某个地方尝试将字符串和元组相加,但这是不允许的。
你可以检查一下代码中是否存在这样的情况,如果有,需要将元组转换为字符串,然后再与字符串相加。例如,如果你想将一个元组 `(1, 2)` 和一个字符串 `"hello"` 相加,你可以这样做:
```python
t = (1, 2)
s = "hello"
result = s + str(t)
```
这里使用 `str()` 函数将元组转换为字符串,然后再将两个字符串相加,得到一个新的字符串 `hello(1, 2)`。
需要注意的是,将元组转换为字符串时,可能需要根据具体的需求进行格式化。在上面的例子中,元组被转换为了一个形如 `(1, 2)` 的字符串,但在实际应用中可能需要根据具体需求进行更复杂的格式化。
阅读全文
相关推荐
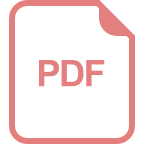
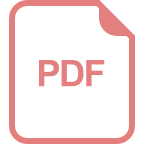















