User : In member function ‘virtual bool IMUFactor::Evaluate(const double* const*, double*, double**) const’: /home/yang/vins-mono-catkin_ws/src/vins-mono-master/vins_estimator/src/utility/../initial/../factor/imu_factor.h:93:18: error: ‘imu_file’ was not
时间: 2023-12-20 20:06:43 浏览: 36
C知道: 这个错误提示表明在函数“IMUFactor::Evaluate(const double* const*, double*, double**)”中使用的变量“imu_file”未定义。要解决这个错误,你需要确保在函数的上下文中正确定义和初始化“imu_file”变量。请检查你的代码,确保在使用该变量之前已经正确定义并赋值。
相关问题
double EvaluateExpression(string str) {//算术表达式求值的算符优先算法 /**************begin************/ /***************end*************/ }生成代码
这是一个要求使用算术表达式的算符优先算法(Operator Precedence Parsing)来计算double类型值的函数。在这个函数中,`str` 参数是一个字符串,表示一个算术表达式,比如 "2 + 3 * 4"。为了生成这个函数的具体代码,我们需要遵循以下步骤:
1. 定义一个栈来存储操作数。
2. 从左到右遍历输入的表达式。
3. 如果遇到数字,直接压入栈中。
4. 遇到运算符时,检查优先级并执行相应的操作:
- 如果是左结合的运算符,将其压入栈。
- 如果是右结合的运算符,弹出栈顶直到遇到左结合的运算符或栈为空,然后进行计算并压回结果。
5. 遍历结束后,栈中只剩下一个元素,就是表达式的结果。
这是一个简化版的伪代码实现:
```cpp
#include <stack>
#include <string>
double EvaluateExpression(const std::string &str) {
std::stack<double> operands;
double num = 0.0;
bool isOperator = false;
char op;
for (char c : str) {
if (isdigit(c)) {
num = num * 10 + (c - '0');
} else {
if (isOperator) {
operands.push(num);
num = 0.0;
isOperator = false;
}
op = c;
if (op == '+' || op == '-') { // 优先级较低的加减
while (!operands.empty() && (operands.top() != '(' || !isOperator && (op == '+' && operands.top() > 0 || op == '-' && operands.top() < 0))) {
num = operands.top();
operands.pop();
isOperator = true;
}
} else if (op == '*' || op == '/') { // 优先级较高的乘除
while (!operands.empty() && (op == '*' || op == '/') && operands.top() != '(') {
num = operands.top();
operands.pop();
operands.push(EvaluateOperation(num, op)); // 递归处理括号内的表达式
isOperator = true;
}
}
}
}
// 处理可能剩下的最后一个操作数
if (isOperator) {
operands.push(num);
}
return EvaluateOperation(operands.top(), '+'); // 结束时,栈顶是最终结果
}
double EvaluateOperation(double a, char op) {
switch (op) {
case '+': return a + operands.top();
case '-': return a - operands.top();
case '*': return a * operands.top();
case '/': return a / operands.top();
default: throw std::invalid_argument("Invalid operator");
}
}
```
const int N = 15; // 棋盘大小 const int M = 6; // 连续棋子数 int currBotColor; // 本方所执子颜色(1为黑,-1为白,棋盘状态亦同) int gridInfo[N][N] = { 0 }; // 先x后y,记录棋盘状态 int dx[] = { -1,-1,-1,0,0,1,1,1 }; int dy[] = { -1,0,1,-1,1,-1,0,1 };int evaluate(); int main() { int x0, y0, x1, y1; // 分析自己收到的输入和自己过往的输出,并恢复棋盘状态 int turnID; cin >> turnID; currBotColor = grid_white; // 先假设自己是白方 for (int i = 0; i < turnID; i++) { // 根据这些输入输出逐渐恢复状态到当前回合 cin >> x0 >> y0 >> x1 >> y1; if (x0 == -1) currBotColor = grid_black; // 第一回合收到坐标是-1, -1,说明我是黑方 if (x0 >= 0) ProcStep(x0, y0, x1, y1, -currBotColor, false); // 模拟对方落子 if (i < turnID - 1) { cin >> x0 >> y0 >> x1 >> y1; if (x0 >= 0) ProcStep(x0, y0, x1, y1, currBotColor, false); // 模拟己方落子 } } /************************************************************************************/ /***在下面填充你的代码,决策结果(本方将落子的位置)存入startX、startY、resultX、resultY中*****/ //下面仅为随机策略的示例代码,且效率低,可删除 int startX, startY, resultX, resultY; bool selfFirstBlack = (turnID == 1 && currBotColor == grid_black);//本方是黑方先手 if(selfFirstBlack){ } else{ } } } /****在上方填充你的代码,决策结果(本方将落子的位置)存入startX、startY、resultX、resultY中****/ /************************************************************************************/ // 决策结束,向平台输出决策结果 cout << startX << ' ' << startY << ' ' << resultX << ' ' << resultY << endl; return 0; }完善主函数并提供博弈树和剪枝代码
以下是一个简单的博弈树搜索和α-β剪枝的示例代码,供参考:
```c++
const int N = 15; // 棋盘大小
const int M = 6; // 连续棋子数
int currBotColor; // 本方所执子颜色(1为黑,-1为白,棋盘状态亦同)
int gridInfo[N][N] = { 0 }; // 先x后y,记录棋盘状态
int dx[] = { -1,-1,-1,0,0,1,1,1 };
int dy[] = { -1,0,1,-1,1,-1,0,1 };
int evaluate() {
// TODO: 根据当前棋盘状态计算评估分数
return 0;
}
int dfs(int depth, int alpha, int beta) {
if (depth == 0) {
return evaluate();
}
int bestScore = -INF;
vector<pair<int, int>> moves;
// 生成所有可行的落子位置
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (gridInfo[i][j] == 0) {
moves.push_back(make_pair(i, j));
}
}
}
// 对可行位置进行排序,以加速剪枝
sort(moves.begin(), moves.end(), [](const pair<int, int>& a, const pair<int, int>& b) {
return evaluate(a.first, a.second) > evaluate(b.first, b.second);
});
// 依次尝试每个可行位置
for (auto& move : moves) {
int x = move.first;
int y = move.second;
gridInfo[x][y] = currBotColor;
currBotColor = -currBotColor;
int score = -dfs(depth - 1, -beta, -alpha);
currBotColor = -currBotColor;
gridInfo[x][y] = 0;
if (score > bestScore) {
bestScore = score;
if (depth == MAX_DEPTH) {
startX = -1;
startY = -1;
resultX = x;
resultY = y;
}
}
alpha = max(alpha, score);
if (alpha >= beta) {
break;
}
}
return bestScore;
}
int main() {
// 初始化棋盘状态和本方颜色
// ...
// 进行博弈树搜索,并使用α-β剪枝
int score = dfs(MAX_DEPTH, -INF, INF);
// 输出决策结果
// ...
return 0;
}
```
其中,`MAX_DEPTH`表示搜索的最大深度,`INF`表示正无穷大,`evaluate`函数用于计算当前棋盘状态的评估分数。
相关推荐
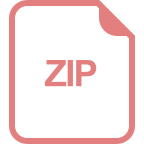
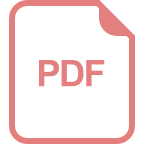
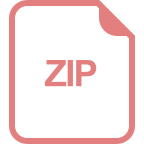












