C++写黑白棋,要求实现绘制6*6棋盘,玩家对战和人机对战
时间: 2024-02-03 08:12:17 浏览: 93
这个任务可以分为以下几个部分:
1. 绘制棋盘:可以使用C++中的图形库或者其他的绘图工具来实现,在这里我以Windows下的GDI+为例,代码如下:
```c++
#include <windows.h>
#include <gdiplus.h>
#pragma comment(lib,"gdiplus.lib")
using namespace Gdiplus;
void DrawBoard(HDC hdc, int nBoardSize, int nCellSize)
{
Graphics graphics(hdc);
Pen pen(Color::Black, 2.0f);
// 绘制棋盘边框
graphics.DrawRectangle(&pen, 0, 0, nBoardSize * nCellSize, nBoardSize * nCellSize);
// 绘制横线和竖线
for (int i = 0; i < nBoardSize; i++) {
graphics.DrawLine(&pen, 0, i * nCellSize, nBoardSize * nCellSize, i * nCellSize);
graphics.DrawLine(&pen, i * nCellSize, 0, i * nCellSize, nBoardSize * nCellSize);
}
}
```
在主函数中调用DrawBoard函数即可绘制棋盘。
2. 玩家对战:可以使用控制台窗口来进行输入和输出。首先定义一个二维数组来表示棋盘,1表示黑棋,2表示白棋,0表示空位。然后写一个函数来判断当前局面是否存在可以落子的位置,如果存在则提示当前玩家输入坐标来落子。每次落子后判断游戏是否结束,如果结束则输出胜利者并退出程序。
```c++
void PlayerVSPlayer()
{
const int nBoardSize = 6;
const int nCellSize = 50;
int board[nBoardSize][nBoardSize] = { 0 }; // 初始化棋盘
HDC hdc = GetDC(NULL);
DrawBoard(hdc, nBoardSize, nCellSize);
int nTurn = 1; // 黑棋先手
while (true) {
bool bHasValidMove = false;
// 判断当前局面是否存在可以落子的位置
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 0 && IsValidMove(board, i, j, nTurn)) {
bHasValidMove = true;
break;
}
}
if (bHasValidMove) break;
}
if (!bHasValidMove) {
int nBlackCount = 0, nWhiteCount = 0;
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 1) nBlackCount++;
else if (board[i][j] == 2) nWhiteCount++;
}
}
if (nBlackCount > nWhiteCount) {
cout << "Black wins!" << endl;
} else if (nWhiteCount > nBlackCount) {
cout << "White wins!" << endl;
} else {
cout << "Draw game!" << endl;
}
break;
}
cout << (nTurn == 1 ? "Black" : "White") << "'s turn:" << endl;
int x, y;
cin >> x >> y;
if (!IsValidMove(board, x, y, nTurn)) {
cout << "Invalid move! Please try again." << endl;
continue;
}
board[x][y] = nTurn;
DrawChess(hdc, x, y, nTurn, nCellSize);
// 判断游戏是否结束
if (IsGameOver(board)) {
int nBlackCount = 0, nWhiteCount = 0;
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 1) nBlackCount++;
else if (board[i][j] == 2) nWhiteCount++;
}
}
if (nBlackCount > nWhiteCount) {
cout << "Black wins!" << endl;
} else if (nWhiteCount > nBlackCount) {
cout << "White wins!" << endl;
} else {
cout << "Draw game!" << endl;
}
break;
}
nTurn = 3 - nTurn; // 切换玩家
}
ReleaseDC(NULL, hdc);
}
```
3. 人机对战:可以使用简单的搜索算法来实现。首先定义一个估值函数来评估当前局面的优劣,然后写一个MinMax算法来搜索最优的下法。具体实现方法可以参考以下代码:
```c++
void PlayerVSComputer()
{
const int nBoardSize = 6;
const int nCellSize = 50;
int board[nBoardSize][nBoardSize] = { 0 }; // 初始化棋盘
HDC hdc = GetDC(NULL);
DrawBoard(hdc, nBoardSize, nCellSize);
int nTurn = 1; // 玩家先手
while (true) {
bool bHasValidMove = false;
// 判断当前局面是否存在可以落子的位置
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 0 && IsValidMove(board, i, j, nTurn)) {
bHasValidMove = true;
break;
}
}
if (bHasValidMove) break;
}
if (!bHasValidMove) {
int nBlackCount = 0, nWhiteCount = 0;
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 1) nBlackCount++;
else if (board[i][j] == 2) nWhiteCount++;
}
}
if (nTurn == 1) {
if (nBlackCount > nWhiteCount) {
cout << "You win!" << endl;
} else if (nWhiteCount > nBlackCount) {
cout << "Computer wins!" << endl;
} else {
cout << "Draw game!" << endl;
}
} else {
if (nWhiteCount > nBlackCount) {
cout << "You win!" << endl;
} else if (nBlackCount > nWhiteCount) {
cout << "Computer wins!" << endl;
} else {
cout << "Draw game!" << endl;
}
}
break;
}
if (nTurn == 1) {
cout << "Your turn:" << endl;
int x, y;
cin >> x >> y;
if (!IsValidMove(board, x, y, nTurn)) {
cout << "Invalid move! Please try again." << endl;
continue;
}
board[x][y] = nTurn;
DrawChess(hdc, x, y, nTurn, nCellSize);
} else {
cout << "Computer's turn:" << endl;
int x, y;
MinMax(board, nTurn, x, y);
board[x][y] = nTurn;
DrawChess(hdc, x, y, nTurn, nCellSize);
}
// 判断游戏是否结束
if (IsGameOver(board)) {
int nBlackCount = 0, nWhiteCount = 0;
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 1) nBlackCount++;
else if (board[i][j] == 2) nWhiteCount++;
}
}
if (nTurn == 1) {
if (nBlackCount > nWhiteCount) {
cout << "You win!" << endl;
} else if (nWhiteCount > nBlackCount) {
cout << "Computer wins!" << endl;
} else {
cout << "Draw game!" << endl;
}
} else {
if (nWhiteCount > nBlackCount) {
cout << "You win!" << endl;
} else if (nBlackCount > nWhiteCount) {
cout << "Computer wins!" << endl;
} else {
cout << "Draw game!" << endl;
}
}
break;
}
nTurn = 3 - nTurn; // 切换玩家
}
ReleaseDC(NULL, hdc);
}
```
其中,IsValidMove函数用来判断当前局面下(x, y)位置是否可以落子,IsGameOver函数用来判断游戏是否结束,DrawChess函数用来绘制棋子。MinMax函数是核心部分,用来搜索最优的下法。具体实现方法可以参考以下代码:
```c++
int Evaluate(int board[][6], int nTurn)
{
const int nBoardSize = 6;
int nBlackCount = 0, nWhiteCount = 0;
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 1) nBlackCount++;
else if (board[i][j] == 2) nWhiteCount++;
}
}
if (nTurn == 1) {
return nBlackCount - nWhiteCount;
} else {
return nWhiteCount - nBlackCount;
}
}
int MinMax(int board[][6], int nTurn, int& x, int& y, int nDepth)
{
const int nBoardSize = 6;
int nValue;
if (nDepth == 0 || IsGameOver(board)) {
return Evaluate(board, nTurn);
}
if (nTurn == 1) {
nValue = INT_MIN;
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 0 && IsValidMove(board, i, j, nTurn)) {
board[i][j] = nTurn;
int nChildValue = MinMax(board, 3 - nTurn, x, y, nDepth - 1);
if (nChildValue > nValue) {
nValue = nChildValue;
x = i;
y = j;
}
board[i][j] = 0;
}
}
}
} else {
nValue = INT_MAX;
for (int i = 0; i < nBoardSize; i++) {
for (int j = 0; j < nBoardSize; j++) {
if (board[i][j] == 0 && IsValidMove(board, i, j, nTurn)) {
board[i][j] = nTurn;
int nChildValue = MinMax(board, 3 - nTurn, x, y, nDepth - 1);
if (nChildValue < nValue) {
nValue = nChildValue;
x = i;
y = j;
}
board[i][j] = 0;
}
}
}
}
return nValue;
}
void MinMax(int board[][6], int nTurn, int& x, int& y)
{
const int nMaxDepth = 4;
MinMax(board, nTurn, x, y, nMaxDepth);
}
```
注意,MinMax函数需要递归调用自身来搜索子节点。在搜索过程中需要注意切换玩家,以及对棋盘状态进行恢复。
以上就是C++实现黑白棋的基本思路,可以根据实际情况进行修改和完善。
阅读全文
相关推荐






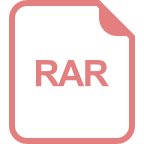



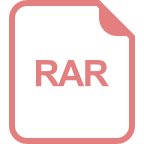


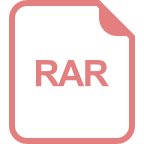

