C++ 写黑白棋,要求绘制6*6的棋盘,检测玩家的所有走法,人机对弈
时间: 2023-11-14 15:04:22 浏览: 136
下面是一个简单的C++黑白棋游戏代码示例,包括绘制棋盘、检测玩家走法和人机对弈。
```c++
#include <iostream>
#include <cstring>
using namespace std;
const int N = 6; // 棋盘大小
const char EMPTY = '-'; // 空格
const char BLACK = 'X'; // 黑色棋子
const char WHITE = 'O'; // 白色棋子
char board[N][N]; // 棋盘
bool turn = true; // true 表示黑棋,false 表示白棋
// 初始化棋盘
void init() {
for (int i = 0; i < N; i++)
for (int j = 0; j < N; j++)
board[i][j] = EMPTY;
board[N/2-1][N/2-1] = WHITE;
board[N/2][N/2] = WHITE;
board[N/2-1][N/2] = BLACK;
board[N/2][N/2-1] = BLACK;
}
// 输出棋盘
void print_board() {
cout << " ";
for (int i = 0; i < N; i++) cout << i+1 << " ";
cout << endl;
for (int i = 0; i < N; i++) {
cout << i+1 << " ";
for (int j = 0; j < N; j++)
cout << board[i][j] << " ";
cout << endl;
}
}
// 判断是否能落子
bool is_valid(int x, int y) {
if (board[x][y] != EMPTY) return false;
char cur = turn ? BLACK : WHITE;
char opp = turn ? WHITE : BLACK;
int dx[8] = {-1, -1, 0, 1, 1, 1, 0, -1};
int dy[8] = {0, 1, 1, 1, 0, -1, -1, -1};
bool flag = false;
for (int i = 0; i < 8; i++) {
int nx = x + dx[i], ny = y + dy[i];
if (nx < 0 || nx >= N || ny < 0 || ny >= N) continue;
if (board[nx][ny] != opp) continue;
while (nx >= 0 && nx < N && ny >= 0 && ny < N && board[nx][ny] == opp) {
nx += dx[i], ny += dy[i];
}
if (nx >= 0 && nx < N && ny >= 0 && ny < N && board[nx][ny] == cur) {
flag = true;
break;
}
}
return flag;
}
// 落子
void place(int x, int y) {
char cur = turn ? BLACK : WHITE;
char opp = turn ? WHITE : BLACK;
board[x][y] = cur;
int dx[8] = {-1, -1, 0, 1, 1, 1, 0, -1};
int dy[8] = {0, 1, 1, 1, 0, -1, -1, -1};
for (int i = 0; i < 8; i++) {
int nx = x + dx[i], ny = y + dy[i];
if (nx < 0 || nx >= N || ny < 0 || ny >= N) continue;
if (board[nx][ny] != opp) continue;
int tx = nx, ty = ny;
while (tx >= 0 && tx < N && ty >= 0 && ty < N && board[tx][ty] == opp) {
tx += dx[i], ty += dy[i];
}
if (tx >= 0 && tx < N && ty >= 0 && ty < N && board[tx][ty] == cur) {
while (tx != x || ty != y) {
board[tx][ty] = cur;
tx -= dx[i], ty -= dy[i];
}
}
}
}
// 判断游戏是否结束
bool is_game_over() {
int cnt1 = 0, cnt2 = 0;
for (int i = 0; i < N; i++)
for (int j = 0; j < N; j++)
if (board[i][j] == BLACK) cnt1++;
else if (board[i][j] == WHITE) cnt2++;
return cnt1 == 0 || cnt2 == 0 || cnt1 + cnt2 == N*N;
}
// 获取当前棋盘上黑棋和白棋的数目
void get_score(int& score1, int& score2) {
score1 = score2 = 0;
for (int i = 0; i < N; i++)
for (int j = 0; j < N; j++)
if (board[i][j] == BLACK) score1++;
else if (board[i][j] == WHITE) score2++;
}
// AI落子
void ai_place() {
int score = -1, x = -1, y = -1;
for (int i = 0; i < N; i++)
for (int j = 0; j < N; j++)
if (is_valid(i, j)) {
char cur = turn ? BLACK : WHITE;
char opp = turn ? WHITE : BLACK;
int dx[8] = {-1, -1, 0, 1, 1, 1, 0, -1};
int dy[8] = {0, 1, 1, 1, 0, -1, -1, -1};
int cnt = 0;
for (int k = 0; k < 8; k++) {
int nx = i + dx[k], ny = j + dy[k];
if (nx < 0 || nx >= N || ny < 0 || ny >= N) continue;
if (board[nx][ny] == opp) {
while (nx >= 0 && nx < N && ny >= 0 && ny < N && board[nx][ny] == opp) {
nx += dx[k], ny += dy[k];
}
if (nx >= 0 && nx < N && ny >= 0 && ny < N && board[nx][ny] == cur) {
while (nx != i || ny != j) {
cnt++;
nx -= dx[k], ny -= dy[k];
}
}
}
}
if (cnt > score) {
score = cnt;
x = i, y = j;
}
}
if (x != -1 && y != -1) place(x, y);
}
int main() {
init();
print_board();
while (!is_game_over()) {
if (turn) cout << "Black's turn: ";
else cout << "White's turn: ";
if (turn) {
bool flag = false;
for (int i = 0; i < N; i++)
for (int j = 0; j < N; j++)
if (is_valid(i, j)) {
flag = true;
break;
}
if (!flag) {
turn = !turn;
continue;
}
int x, y;
cin >> x >> y;
x--, y--;
if (is_valid(x, y)) {
place(x, y);
} else {
cout << "Invalid move!" << endl;
continue;
}
} else {
ai_place();
}
print_board();
int score1, score2;
get_score(score1, score2);
cout << "Black: " << score1 << ", White: " << score2 << endl;
turn = !turn;
}
int score1, score2;
get_score(score1, score2);
if (score1 > score2) cout << "Black wins!" << endl;
else if (score1 < score2) cout << "White wins!" << endl;
else cout << "Draw!" << endl;
return 0;
}
```
该代码实现了基本的黑白棋游戏功能,包括绘制棋盘、检测玩家走法和人机对弈。玩家通过输入坐标来落子,AI通过搜索所有合法落子来选择最优的位置。
阅读全文
相关推荐

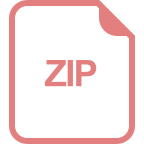
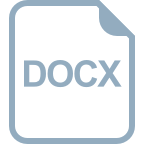
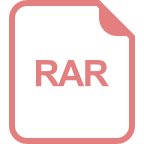
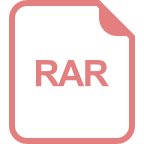
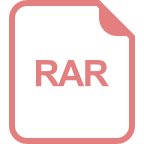
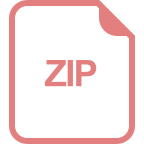
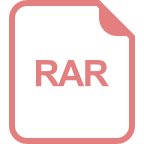
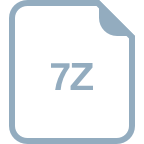
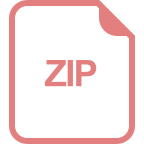
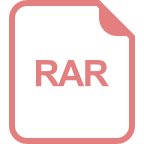
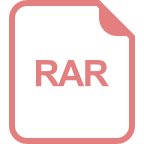
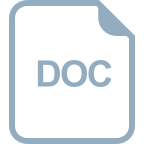
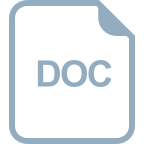
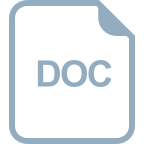
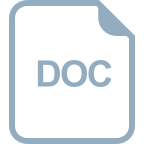