C++写黑白棋,要求实现绘制6*6棋盘,玩家对战和人机对战两种
时间: 2024-02-03 08:12:18 浏览: 120
好的,下面是一个简单的黑白棋游戏的实现,包含了绘制棋盘、玩家对战和人机对战两种模式。代码使用了C++11标准。
```
#include <iostream>
#include <vector>
using namespace std;
const int BOARD_SIZE = 6; // 棋盘大小
enum class Player {
BLACK,
WHITE
};
enum class CellStatus {
EMPTY,
BLACK,
WHITE
};
class Board {
public:
Board() {
cells_.resize(BOARD_SIZE, vector<CellStatus>(BOARD_SIZE, CellStatus::EMPTY));
// 初始化棋盘中间的四个棋子
cells_[2][2] = cells_[3][3] = CellStatus::BLACK;
cells_[2][3] = cells_[3][2] = CellStatus::WHITE;
}
// 绘制棋盘
void DrawBoard() {
cout << " ";
for (int i = 0; i < BOARD_SIZE; ++i) {
cout << i << " ";
}
cout << endl;
for (int i = 0; i < BOARD_SIZE; ++i) {
cout << i << " ";
for (int j = 0; j < BOARD_SIZE; ++j) {
switch (cells_[i][j]) {
case CellStatus::EMPTY:
cout << "+ ";
break;
case CellStatus::BLACK:
cout << "X ";
break;
case CellStatus::WHITE:
cout << "O ";
break;
default:
break;
}
}
cout << endl;
}
}
// 判断落子是否合法
bool IsValidMove(int x, int y, Player player) {
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE) {
return false;
}
if (cells_[x][y] != CellStatus::EMPTY) {
return false;
}
// 判断落子是否能够翻转对手的棋子
CellStatus cur_status = (player == Player::BLACK) ? CellStatus::BLACK : CellStatus::WHITE;
CellStatus oppo_status = (player == Player::BLACK) ? CellStatus::WHITE : CellStatus::BLACK;
bool found = false;
for (int dx = -1; dx <= 1; ++dx) {
for (int dy = -1; dy <= 1; ++dy) {
if (dx == 0 && dy == 0) {
continue;
}
int nx = x + dx, ny = y + dy;
while (nx >= 0 && nx < BOARD_SIZE && ny >= 0 && ny < BOARD_SIZE && cells_[nx][ny] == oppo_status) {
nx += dx;
ny += dy;
if (nx >= 0 && nx < BOARD_SIZE && ny >= 0 && ny < BOARD_SIZE && cells_[nx][ny] == cur_status) {
found = true;
break;
}
}
}
if (found) {
break;
}
}
return found;
}
// 落子
void PlacePiece(int x, int y, Player player) {
CellStatus cur_status = (player == Player::BLACK) ? CellStatus::BLACK : CellStatus::WHITE;
CellStatus oppo_status = (player == Player::BLACK) ? CellStatus::WHITE : CellStatus::BLACK;
cells_[x][y] = cur_status;
// 翻转对手的棋子
for (int dx = -1; dx <= 1; ++dx) {
for (int dy = -1; dy <= 1; ++dy) {
if (dx == 0 && dy == 0) {
continue;
}
int nx = x + dx, ny = y + dy;
bool found = false;
while (nx >= 0 && nx < BOARD_SIZE && ny >= 0 && ny < BOARD_SIZE && cells_[nx][ny] == oppo_status) {
nx += dx;
ny += dy;
if (nx >= 0 && nx < BOARD_SIZE && ny >= 0 && ny < BOARD_SIZE && cells_[nx][ny] == cur_status) {
found = true;
break;
}
}
if (found) {
nx -= dx;
ny -= dy;
while (nx != x || ny != y) {
cells_[nx][ny] = cur_status;
nx -= dx;
ny -= dy;
}
}
}
}
}
// 判断游戏是否结束
bool IsGameOver() {
int black_count = 0, white_count = 0;
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
if (cells_[i][j] == CellStatus::BLACK) {
++black_count;
} else if (cells_[i][j] == CellStatus::WHITE) {
++white_count;
}
}
}
return (black_count == 0 || white_count == 0 || black_count + white_count == BOARD_SIZE * BOARD_SIZE);
}
// 获取黑子数量
int GetBlackCount() {
int count = 0;
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
if (cells_[i][j] == CellStatus::BLACK) {
++count;
}
}
}
return count;
}
// 获取白子数量
int GetWhiteCount() {
int count = 0;
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
if (cells_[i][j] == CellStatus::WHITE) {
++count;
}
}
}
return count;
}
private:
vector<vector<CellStatus>> cells_;
};
// 玩家对战模式
void PlayerVsPlayer() {
Board board;
Player cur_player = Player::BLACK;
while (!board.IsGameOver()) {
board.DrawBoard();
cout << "Player " << ((cur_player == Player::BLACK) ? "X" : "O") << "'s turn." << endl;
int x, y;
do {
cout << "Enter x and y coordinates: ";
cin >> x >> y;
} while (!board.IsValidMove(x, y, cur_player));
board.PlacePiece(x, y, cur_player);
cur_player = (cur_player == Player::BLACK) ? Player::WHITE : Player::BLACK;
}
board.DrawBoard();
int black_count = board.GetBlackCount(), white_count = board.GetWhiteCount();
if (black_count > white_count) {
cout << "Player X wins! (Black: " << black_count << ", White: " << white_count << ")" << endl;
} else if (white_count > black_count) {
cout << "Player O wins! (Black: " << black_count << ", White: " << white_count << ")" << endl;
} else {
cout << "It's a tie! (Black: " << black_count << ", White: " << white_count << ")" << endl;
}
}
// 人机对战模式
void PlayerVsComputer() {
Board board;
Player cur_player = Player::BLACK;
while (!board.IsGameOver()) {
board.DrawBoard();
if (cur_player == Player::BLACK) {
cout << "Player X's turn." << endl;
int x, y;
do {
cout << "Enter x and y coordinates: ";
cin >> x >> y;
} while (!board.IsValidMove(x, y, cur_player));
board.PlacePiece(x, y, cur_player);
} else {
cout << "Computer's turn." << endl;
int best_x = -1, best_y = -1, best_score = -1;
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
if (board.IsValidMove(i, j, cur_player)) {
Board tmp_board = board;
tmp_board.PlacePiece(i, j, cur_player);
int score = tmp_board.GetBlackCount() - tmp_board.GetWhiteCount();
if (score > best_score) {
best_score = score;
best_x = i;
best_y = j;
}
}
}
}
board.PlacePiece(best_x, best_y, cur_player);
}
cur_player = (cur_player == Player::BLACK) ? Player::WHITE : Player::BLACK;
}
board.DrawBoard();
int black_count = board.GetBlackCount(), white_count = board.GetWhiteCount();
if (black_count > white_count) {
cout << "Player X wins! (Black: " << black_count << ", White: " << white_count << ")" << endl;
} else if (white_count > black_count) {
cout << "Computer wins! (Black: " << black_count << ", White: " << white_count << ")" << endl;
} else {
cout << "It's a tie! (Black: " << black_count << ", White: " << white_count << ")" << endl;
}
}
int main() {
int mode;
do {
cout << "Choose mode: 1 for player vs. player, 2 for player vs. computer: ";
cin >> mode;
} while (mode != 1 && mode != 2);
if (mode == 1) {
PlayerVsPlayer();
} else {
PlayerVsComputer();
}
return 0;
}
```
这个实现中使用了一个 `Board` 类来表示棋盘,并且提供了一系列方法来实现落子、判断游戏是否结束等功能。`PlayerVsPlayer` 和 `PlayerVsComputer` 分别表示玩家对战和人机对战模式。在人机对战模式中,我们使用了一个简单的启发式算法来选择电脑的最佳落子位置,即选择使己方棋子数量减去对方棋子数量最大的位置。
阅读全文
相关推荐










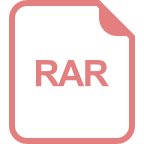


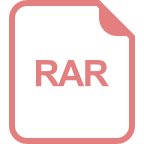

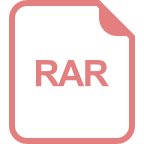
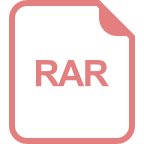

