写C++代码将YOLOV5返回多个类坐标存节点用A星寻路
时间: 2024-01-26 19:05:08 浏览: 107
好的,我可以为您提供一些参考代码来完成这个任务。首先,我们需要将YOLOV5返回的多个类坐标存储在节点中,这可以通过以下代码实现:
```c++
struct Node {
int x;
int y;
vector<float> classes;
};
vector<Node> nodes;
// 调用YOLOV5并将返回的坐标和类存储在节点中
void getNodes() {
// 调用YOLOV5并返回坐标和类
vector<pair<pair<int, int>, vector<float>>> results = callYOLOV5();
// 将坐标和类存储在节点中
for (auto result : results) {
Node node;
node.x = result.first.first;
node.y = result.first.second;
node.classes = result.second;
nodes.push_back(node);
}
}
```
接下来,我们可以使用A*算法来寻路。以下是一个简单的A*算法实现:
```c++
struct Point {
int x;
int y;
};
// 计算两点之间的曼哈顿距离
int manhattanDistance(Point a, Point b) {
return abs(a.x - b.x) + abs(a.y - b.y);
}
// A*搜索算法
vector<Point> AStarSearch(Point start, Point end) {
map<Point, Point> cameFrom;
map<Point, int> costSoFar;
priority_queue<pair<int, Point>, vector<pair<int, Point>>, greater<pair<int, Point>>> frontier;
frontier.push(make_pair(0, start));
cameFrom[start] = start;
costSoFar[start] = 0;
while (!frontier.empty()) {
Point current = frontier.top().second;
frontier.pop();
if (current.x == end.x && current.y == end.y) {
break;
}
vector<Point> neighbors = getNeighbors(current);
for (Point next : neighbors) {
int newCost = costSoFar[current] + manhattanDistance(current, next);
if (!costSoFar.count(next) || newCost < costSoFar[next]) {
costSoFar[next] = newCost;
int priority = newCost + manhattanDistance(next, end);
frontier.push(make_pair(priority, next));
cameFrom[next] = current;
}
}
}
vector<Point> path;
Point current = end;
while (current.x != start.x || current.y != start.y) {
path.push_back(current);
current = cameFrom[current];
}
path.push_back(start);
reverse(path.begin(), path.end());
return path;
}
```
在上面的代码中,我们使用了一个结构体 `Point` 表示一个节点的坐标。 `getNeighbors` 函数返回一个节点的邻居节点列表。 `AStarSearch` 函数返回从起点到终点的最短路径。
那么将YOLOV5返回的多个类坐标存储到节点中,并使用A*算法进行寻路的完整代码如下:
```c++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int x;
int y;
vector<float> classes;
};
vector<Node> nodes;
struct Point {
int x;
int y;
};
// 计算两点之间的曼哈顿距离
int manhattanDistance(Point a, Point b) {
return abs(a.x - b.x) + abs(a.y - b.y);
}
// A*搜索算法
vector<Point> AStarSearch(Point start, Point end) {
map<Point, Point> cameFrom;
map<Point, int> costSoFar;
priority_queue<pair<int, Point>, vector<pair<int, Point>>, greater<pair<int, Point>>> frontier;
frontier.push(make_pair(0, start));
cameFrom[start] = start;
costSoFar[start] = 0;
while (!frontier.empty()) {
Point current = frontier.top().second;
frontier.pop();
if (current.x == end.x && current.y == end.y) {
break;
}
vector<Point> neighbors = getNeighbors(current);
for (Point next : neighbors) {
int newCost = costSoFar[current] + manhattanDistance(current, next);
if (!costSoFar.count(next) || newCost < costSoFar[next]) {
costSoFar[next] = newCost;
int priority = newCost + manhattanDistance(next, end);
frontier.push(make_pair(priority, next));
cameFrom[next] = current;
}
}
}
vector<Point> path;
Point current = end;
while (current.x != start.x || current.y != start.y) {
path.push_back(current);
current = cameFrom[current];
}
path.push_back(start);
reverse(path.begin(), path.end());
return path;
}
// 调用YOLOV5并将返回的坐标和类存储在节点中
void getNodes() {
// 调用YOLOV5并返回坐标和类
vector<pair<pair<int, int>, vector<float>>> results = callYOLOV5();
// 将坐标和类存储在节点中
for (auto result : results) {
Node node;
node.x = result.first.first;
node.y = result.first.second;
node.classes = result.second;
nodes.push_back(node);
}
}
// 获取一个节点的邻居节点列表
vector<Point> getNeighbors(Point node) {
vector<Point> neighbors;
for (Node n : nodes) {
if (n.x == node.x && n.y == node.y) {
continue;
}
neighbors.push_back({n.x, n.y});
}
return neighbors;
}
int main() {
// 获取节点
getNodes();
// 设置起点和终点
Point start = {0, 0};
Point end = {10, 10};
// 寻路
vector<Point> path = AStarSearch(start, end);
// 输出路径
for (Point p : path) {
cout << "(" << p.x << ", " << p.y << ") ";
}
cout << endl;
return 0;
}
```
请注意,上面的代码只是一个示例,您需要根据您的实际情况进行修改和调整。
阅读全文
相关推荐
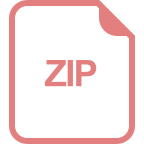




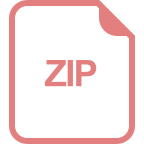
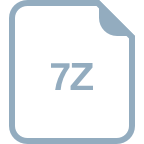
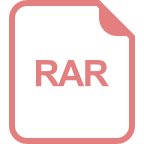
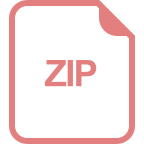
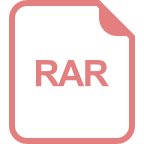
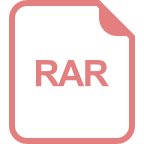
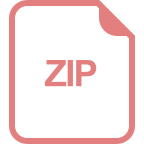
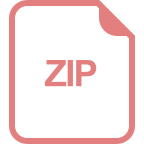
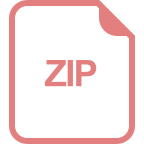
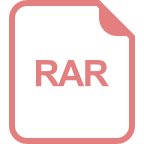
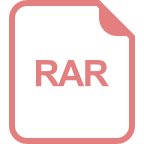
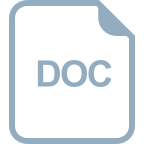