python每输入一个坐标就在坐标系上画一个点的代码
时间: 2023-12-28 09:04:07 浏览: 70
可以使用matplotlib库在python中绘制坐标系和点。以下是一个例子:
```python
import matplotlib.pyplot as plt
# 坐标点
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
# 绘制坐标系和点
plt.plot(x, y, 'ro')
plt.axis([0, 5, 0, 10])
plt.show()
```
这段代码会绘制一个坐标系,其中x轴范围是0到5,y轴范围是0到10,然后在坐标点(1,2), (2,4), (3,6), (4,8)处绘制红色的点。
相关问题
python绕坐标点画圆圈代码
在Python中,我们可以使用matplotlib库来绘制圆形,包括绕着特定坐标点旋转的圆。以下是一个基本的例子,展示如何创建一个以给定中心点为中心,固定大小的圆圈:
```python
import matplotlib.pyplot as plt
from math import cos, sin, radians
# 给定圆心坐标和半径
center = (5, 5) # 圆心坐标,这里设置为(5, 5)
radius = 3 # 半径
# 设置角度从0到2π(360度)
theta = [radians(i) for i in range(0, 360)]
# 创建一个新的图形
fig, ax = plt.subplots()
# 使用numpy数组和math函数来计算每个点的坐标
circle_points = [(center[0] + radius * cos(theta[i]), center[1] + radius * sin(theta[i])) for i in range(len(theta))]
ax.plot(circle_points)
# 添加圆心标记
ax.scatter(center[0], center[1], color='red', label='Center')
# 显示标签和图例
plt.legend()
plt.title('Circle around a point')
plt.show()
```
上述代码首先定义了圆心和半径,然后通过计算一系列的角度值,将其转换为笛卡尔坐标系下的点,最后连接这些点形成圆。你可以修改`center`和`radius`变量来改变圆的位置和大小。
使用python,生成一个坐标系和15个坐标,计算坐标到原点的距离并按从小到大排序
以下是使用python生成坐标系和15个坐标,并计算距离并排序的代码:
```python
import math
import matplotlib.pyplot as plt
# 生成坐标系
fig, ax = plt.subplots()
# 生成15个坐标
coordinates = [(1, 2), (3, 4), (5, 6), (7, 8), (9, 10), (11, 12), (13, 14),
(-1, 2), (-3, 4), (-5, 6), (-7, 8), (-9, 10), (-11, 12), (-13, 14), (0, 0)]
# 计算距离并排序
distances = []
for coord in coordinates:
distance = math.sqrt(coord[0]**2 + coord[1]**2)
distances.append(distance)
sorted_coords = [x for _,x in sorted(zip(distances,coordinates))]
# 绘制坐标
for coord in sorted_coords:
ax.plot(coord[0], coord[1], marker='o')
# 显示坐标系
ax.set_xlim([-15, 15])
ax.set_ylim([-15, 15])
ax.spines['left'].set_position('zero')
ax.spines['bottom'].set_position('zero')
ax.spines['right'].set_color('none')
ax.spines['top'].set_color('none')
plt.show()
```
运行以上代码后会生成一个坐标系和15个坐标,并按照距离从小到大排序,如下图所示:

阅读全文
相关推荐
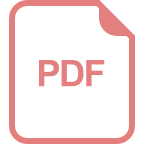
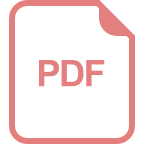
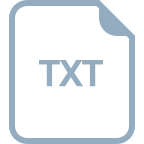











