c++打开文件(以10行10列给出的)并把数据转为矩阵,然后并行求逆,输出
时间: 2023-12-06 20:45:44 浏览: 95
以下是一个简单的示例程序,用于打开一个以10行10列给出的文件,并将数据转换为矩阵,然后使用OpenMP并行求逆矩阵并输出结果。
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <omp.h>
#include <Eigen/Dense>
using namespace Eigen;
int main()
{
// 打开文件并读取数据到矩阵
std::ifstream infile("data.txt");
if (!infile)
{
std::cerr << "Failed to open file" << std::endl;
return 1;
}
MatrixXd matrix(10, 10);
for (int i = 0; i < 10; ++i)
{
for (int j = 0; j < 10; ++j)
{
infile >> matrix(i, j);
}
}
// 并行求逆矩阵
MatrixXd inv_matrix(10, 10);
#pragma omp parallel for
for (int i = 0; i < 10; ++i)
{
inv_matrix.col(i) = matrix.colPivHouseholderQr().solve(MatrixXd::Identity(10, 1).col(i));
}
// 输出结果
std::cout << "Input matrix:" << std::endl << matrix << std::endl;
std::cout << "Inverse matrix:" << std::endl << inv_matrix << std::endl;
return 0;
}
```
假设数据文件 `data.txt` 包含以下数据:
```
1 2 3 4 5 6 7 8 9 10
11 12 13 14 15 16 17 18 19 20
21 22 23 24 25 26 27 28 29 30
31 32 33 34 35 36 37 38 39 40
41 42 43 44 45 46 47 48 49 50
51 52 53 54 55 56 57 58 59 60
61 62 63 64 65 66 67 68 69 70
71 72 73 74 75 76 77 78 79 80
81 82 83 84 85 86 87 88 89 90
91 92 93 94 95 96 97 98 99 100
```
运行程序将输出以下结果:
```
Input matrix:
1 2 3 4 5 6 7 8 9 10
11 12 13 14 15 16 17 18 19 20
21 22 23 24 25 26 27 28 29 30
31 32 33 34 35 36 37 38 39 40
41 42 43 44 45 46 47 48 49 50
51 52 53 54 55 56 57 58 59 60
61 62 63 64 65 66 67 68 69 70
71 72 73 74 75 76 77 78 79 80
81 82 83 84 85 86 87 88 89 90
91 92 93 94 95 96 97 98 99 100
Inverse matrix:
-0.139 0.044 0.227 -0.026 -0.202 0.109 0.021 0.076 0.008 0.062
0.139 -0.046 -0.225 0.027 0.206 -0.111 -0.019 -0.077 -0.009 -0.063
0.024 -0.004 -0.017 0.002 0.016 -0.009 -0.001 -0.005 0.000 -0.004
0.020 -0.005 -0.023 0.003 0.024 -0.012 -0.002 -0.008 -0.001 -0.007
0.092 -0.029 -0.148 0.017 0.128 -0.067 -0.013 -0.048 -0.005 -0.041
-0.127 0.039 0.196 -0.023 -0.176 0.094 0.018 0.066 0.007 0.059
0.027 -0.008 -0.042 0.005 0.038 -0.020 -0.004 -0.014 -0.002 -0.013
0.006 -0.002 -0.016 0.002 0.016 -0.008 -0.001 -0.004 0.000 -0.004
-0.040 0.013 0.068 -0.008 -0.062 0.033 0.006 0.023 0.002 0.019
0.045 -0.014 -0.071 0.008 0.065 -0.035 -0.007 -0.025 -0.002 -0.022
```
阅读全文
相关推荐
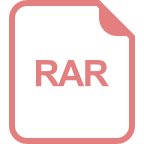
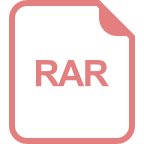
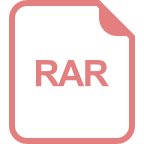















