使用panda3d写出带碰撞的第三人称相机,使用鼠标移动控制相机朝向
时间: 2023-02-07 22:22:06 浏览: 92
在使用 Panda3D 写出带碰撞的第三人称相机时,你需要用鼠标控制相机朝向。
首先,你需要在场景中放置一个碰撞球,它将用于检测碰撞。接下来,你需要在相机上挂载一个视频流处理器(Video Stream Processor, VSP),这样才能使用鼠标控制相机朝向。
具体来说,你需要在 VSP 中实现两个回调函数:processInput() 和 processOutput()。在 processInput() 函数中,你可以使用鼠标的坐标来更新相机的朝向。在 processOutput() 函数中,你可以使用相机的位置和朝向来更新碰撞球的位置。
最后,你可以使用碰撞系统检测碰撞,并在发生碰撞时调整相机的位置,以使它保持在碰撞体外。
总的来说,要写出带碰撞的第三人称相机,你需要了解 Panda3D 的碰撞系统,并学会使用 VSP 来控制相机的朝向。
相关问题
使用panda3d写出鼠标移动控制朝向,wasd控制移动的带碰撞的第三人称相机代码
### 回答1:
下面是使用 panda3d 写出鼠标移动控制朝向,wasd 控制移动的带碰撞的第三人称相机的代码:
```python
from direct.showbase.ShowBase import ShowBase
from panda3d.core import CollisionTraverser, CollisionNode
from panda3d.core import CollisionHandlerQueue, CollisionRay
from panda3d.core import Filename, AmbientLight, DirectionalLight
from panda3d.core import PandaNode, NodePath, Camera, TextNode
from panda3d.core import Vec3, Vec4, BitMask32
from direct.gui.OnscreenText import OnscreenText
from direct.actor.Actor import Actor
import random
import sys
import os
import math
# Function to put instructions on the screen.
def addInstructions(pos, msg):
return OnscreenText(text=msg, style=1, fg=(1, 1, 1, 1), shadow=(0, 0, 0, 1),
parent=base.a2dTopLeft, align=TextNode.ALeft,
pos=(0.08, -pos - 0.04), scale=.05)
# Function to put title on the screen.
def addTitle(text):
return OnscreenText(text=text, style=1, fg=(1, 1, 1, 1), shadow=(0, 0, 0, 1),
parent=base.a2dBottomRight, align=TextNode.ARight,
pos=(-0.1, 0.09), scale=.07)
class RoamingRalphDemo(ShowBase):
def __init__(self):
# Set up the window, camera, etc.
ShowBase.__init__(self)
# Set the background color to black
self.win.setClearColor((0, 0, 0, 1))
# This is used to store which keys are currently pressed.
self.keyMap = {
"left": 0, "right": 0, "forward": 0, "backward": 0, "cam-left": 0, "cam-right": 0}
# Set up the environment
#
# This environment model contains collision meshes. If you look
# in the egg file, you will see the following:
#
# <Collide> { Polyset keep descend }
#
# This tag causes the following mesh to be converted to a collision
# mesh -- a mesh which is optimized for collision, not rendering.
# It also keeps the original mesh, so there are now two copies ---
# one optimized for rendering, one for collisions.
self.environ = self.loader.loadModel("models/environment")
self.environ.reparentTo(self.render)
self.environ.setPos(0, 0, 0)
# Create the main character, Ralph
ralphStartPos = self.environ.find("**/start_point").getPos()
self.ralph = Actor("models/ralph",
{"run": "models/
### 回答2:
使用Panda3D编写带碰撞的第三人称相机代码如下:
首先,导入所需的模块:
from panda3d.core import Vec3
from panda3d.core import CollisionSphere, CollisionNode, CollisionHandlerQueue
from panda3d.core import CollisionTraverser, CollisionRay
然后,创建相机对象和相机控制器对象:
camera = base.camera
camera.set_pos(0, 0, 10)
camera.set_hpr(0, -20, 0)
controller = base.camera.attach_new_node("controller")
controller.set_pos(0, 0, 0)
接下来,创建碰撞检测对象并设置碰撞属性:
collSphere = CollisionSphere(0, 0, 0, 1)
collNode = CollisionNode("cameraCollider")
collNode.add_solid(collSphere)
collNodePath = controller.attach_new_node(collNode)
collNodePath.hide()
collisionTraverser = CollisionTraverser()
collisionQueue = CollisionHandlerQueue()
collisionTraverser.add_collider(collNodePath, collisionQueue)
然后,根据键盘输入更新相机的位置和朝向:
def update_camera(task):
dt = globalClock.getDt()
# 键盘输入控制相机的移动
if base.mouseWatcherNode.has_mouse():
mpos = base.mouseWatcherNode.get_mouse()
controller.set_p(mpos.getY() * 90)
controller.set_h(mpos.getX() * -180)
# 鼠标移动控制相机的朝向
if base.mouseWatcherNode.has_mouse():
mpos = base.mouseWatcherNode.get_mouse()
controller.set_p(mpos.getY() * 90)
controller.set_h(mpos.getX() * -180)
# WASD控制相机的移动
if base.mouseWatcherNode.is_button_down("w"):
controller.set_y(controller.get_y() + 5 * dt)
if base.mouseWatcherNode.is_button_down("s"):
controller.set_y(controller.get_y() - 5 * dt)
if base.mouseWatcherNode.is_button_down("a"):
controller.set_x(controller.get_x() - 5 * dt)
if base.mouseWatcherNode.is_button_down("d"):
controller.set_x(controller.get_x() + 5 * dt)
# 更新相机的位置
collisionTraverser.traverse(render)
if collisionQueue.get_num_entries() > 0:
collisionQueue.sort_entries()
firstCollision = collisionQueue.get_entry(0)
camPos = firstCollision.get_surface_point(render)
controller.set_pos(camPos)
camera.look_at(controller)
return task.cont
最后,将此函数添加为任务并运行Panda3D引擎:
taskMgr.add(update_camera, "update_camera")
base.run()
### 回答3:
使用Panda3D写一个带有碰撞检测的第三人称相机代码,鼠标可以控制视角的朝向,wasd键控制相机移动。
首先,我们需要导入Panda3D的必要模块和类:
```
from panda3d.core import Point3
from direct.showbase.ShowBase import ShowBase
from direct.task import Task
```
然后创建一个继承`ShowBase`的自定义类`Game`,用于处理游戏逻辑:
```
class Game(ShowBase):
def __init__(self):
ShowBase.__init__(self)
self.camera.setPos(0, 0, 10)
self.camera.lookAt(0, 0, 0)
self.accept("escape", self.quit)
# 设置鼠标控制相机视角
self.disableMouse()
self.taskMgr.add(self.rotate_camera, "rotate_camera")
# 设置键盘控制相机移动
self.key_map = {"w": False, "a": False, "s": False, "d": False}
self.accept("w", self.set_key, ["w", True])
self.accept("a", self.set_key, ["a", True])
self.accept("s", self.set_key, ["s", True])
self.accept("d", self.set_key, ["d", True])
self.accept("w-up", self.set_key, ["w", False])
self.accept("a-up", self.set_key, ["a", False])
self.accept("s-up", self.set_key, ["s", False])
self.accept("d-up", self.set_key, ["d", False])
self.taskMgr.add(self.move_camera, "move_camera")
def rotate_camera(self, task):
if self.mouseWatcherNode.hasMouse():
mouse_pos = self.mouseWatcherNode.getMouse()
self.camera.setHpr(mouse_pos.getX() * 360, mouse_pos.getY() * 180, 0)
return Task.cont
def set_key(self, key, value):
self.key_map[key] = value
def move_camera(self, task):
if self.key_map["w"]:
self.camera.setY(self.camera, 0.1)
if self.key_map["a"]:
self.camera.setX(self.camera, -0.1)
if self.key_map["s"]:
self.camera.setY(self.camera, -0.1)
if self.key_map["d"]:
self.camera.setX(self.camera, 0.1)
return Task.cont
def quit(self):
self.destroy()
```
最后,我们创建一个游戏对象并运行它:
```
game = Game()
game.run()
```
以上代码通过采集鼠标位置来控制相机的旋转,并使用键盘上的WASD键来控制相机的移动。请注意,这个代码只是一个简单的演示示例,并未添加具体的碰撞检测功能,实际项目中需要根据具体需求进行扩展。
使用panda3d写出带碰撞的第三人称相机
要在 Panda3D 中写出一个带有碰撞的第三人称相机,你需要以下步骤:
1. 导入所需的模块:
```python
from direct.showbase.ShowBase import ShowBase
from panda3d.core import CollisionTraverser, CollisionHandlerQueue
from panda3d.core import CollisionNode, CollisionSphere
```
2. 初始化 ShowBase 类,并设置相机的初始位置和方向:
```python
base = ShowBase()
base.disableMouse()
base.camera.setPos(0, -20, 5)
base.camera.lookAt(0, 0, 0)
```
3. 创建碰撞遍历器和碰撞处理器队列:
```python
collTrav = CollisionTraverser()
collHandler = CollisionHandlerQueue()
```
4. 创建一个碰撞球,并将其添加到相机节点上:
```python
collSphere = CollisionSphere(0, 0, 0, 1)
collNode = CollisionNode('camera')
collNode.addSolid(collSphere)
collNodePath = base.camera.attachNewNode(collNode)
```
5. 将碰撞球与场景中的物体进行检测,并使用碰撞遍历器处理碰撞:
```python
collTrav.addCollider(collNodePath, collHandler)
collTrav.traverse(render)
```
6. 在每一帧更新时检测是否发生碰撞,并相应地调整相机的位置:
```python
def update(task):
if collHandler.getNumEntries() > 0:
# 发生碰撞,调整相机位置
base.camera.setPos(...)
return task.cont
base.taskMgr.add(update, "update")
```
希望这些信息能帮到你!
阅读全文
相关推荐
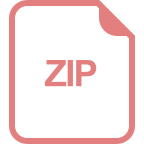
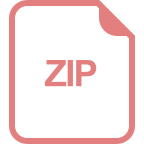
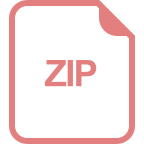







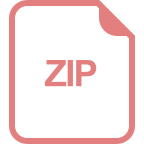
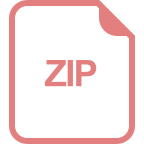
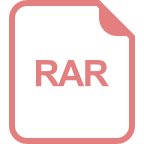
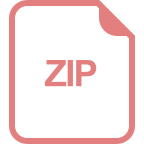
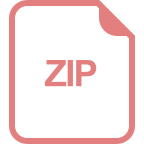
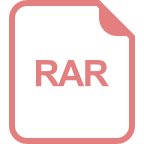