用Verilog写一个电子秒表,包含顶层模块,时钟模块,按键消抖模块,计数模块,译码显示模块
时间: 2023-07-13 14:11:53 浏览: 49
好的,我可以为您提供一个基本的Verilog代码框架,实现一个简单的电子秒表,包括顶层模块、时钟模块、按键消抖模块、计数模块和译码显示模块。请注意,这只是一个示例代码,您需要根据具体需求进行修改和优化。
```
// 顶层模块
module stopwatch(clk, reset, start_stop, display);
input clk, reset, start_stop;
output [6:0] display;
wire [6:0] count;
// 实例化子模块
clock clock_inst(clk, reset);
debounce debounce_inst(start_stop, clk, reset);
counter counter_inst(clk, reset, debounce_inst.output);
decoder decoder_inst(count, display);
// 连接子模块
assign count = counter_inst.count;
endmodule
// 时钟模块
module clock(clk, reset);
input clk, reset;
reg [31:0] count;
always @(posedge clk or posedge reset) begin
if (reset) begin
count <= 0;
end else begin
count <= count + 1;
end
end
endmodule
// 按键消抖模块
module debounce(input start_stop, input clk, input reset, output reg output);
reg [1:0] state;
reg [1:0] next_state;
parameter IDLE = 2'b00;
parameter PRESS = 2'b01;
parameter RELEASE = 2'b10;
parameter DEBOUNCE_TIME = 10; // 消抖时间,单位为时钟周期
always @(posedge clk or posedge reset) begin
if (reset) begin
state <= IDLE;
output <= 1'b0;
end else begin
state <= next_state;
end
end
always @* begin
next_state = state;
case(state)
IDLE: begin
if (start_stop == 1'b0) begin
next_state = PRESS;
end
end
PRESS: begin
if (start_stop == 1'b0) begin
next_state = RELEASE;
end else begin
next_state = IDLE;
end
end
RELEASE: begin
if (start_stop == 1'b0) begin
next_state = PRESS;
output <= 1'b1;
#DEBOUNCE_TIME output <= 1'b0;
end else begin
next_state = IDLE;
end
end
endcase
end
endmodule
// 计数模块
module counter(input clk, input reset, input start_stop, output reg [6:0] count);
reg [31:0] count_reg;
reg [1:0] state;
reg [1:0] next_state;
parameter IDLE = 2'b00;
parameter COUNTING = 2'b01;
parameter STOPPED = 2'b10;
always @(posedge clk or posedge reset) begin
if (reset) begin
count_reg <= 0;
state <= IDLE;
end else begin
state <= next_state;
case(state)
IDLE: begin
if (start_stop == 1'b1) begin
next_state = COUNTING;
end
end
COUNTING: begin
if (start_stop == 1'b0) begin
next_state = STOPPED;
end else begin
count_reg <= count_reg + 1;
next_state = COUNTING;
end
end
STOPPED: begin
if (start_stop == 1'b1) begin
next_state = COUNTING;
end else begin
next_state = STOPPED;
end
end
endcase
end
end
assign count = count_reg[6:0];
endmodule
// 译码显示模块
module decoder(input [6:0] count, output reg [6:0] display);
always @* begin
case(count)
7'h00: display = 7'b1111110;
7'h01: display = 7'b0110000;
7'h02: display = 7'b1101101;
7'h03: display = 7'b1111001;
7'h04: display = 7'b0110011;
7'h05: display = 7'b1011011;
7'h06: display = 7'b1011111;
7'h07: display = 7'b1110000;
7'h08: display = 7'b1111111;
7'h09: display = 7'b1111011;
default: display = 7'b0000000;
endcase
end
endmodule
```
这个代码框架包含了顶层模块、时钟模块、按键消抖模块、计数模块和译码显示模块。您需要使用模拟器进行仿真,并根据需要进行修改和优化。
相关推荐
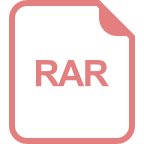














