python 俄罗斯方块代码
时间: 2023-07-23 15:43:08 浏览: 52
好的,以下是一个简单的 Python 俄罗斯方块游戏的代码示例:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏区域大小
block_size = 30
rows = 20
cols = 10
width = block_size * cols
height = block_size * rows
# 颜色定义
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
# 创建游戏区域
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Tetris")
# 方块类型定义
shapes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6],
[6, 6]],
[[7, 7, 7, 7]]
]
# 方块颜色定义
colors = [
white,
blue,
green,
red,
(255, 165, 0),
(128, 0, 128),
(255, 0, 255)
]
# 初始化游戏区域
board = [[0] * cols for _ in range(rows)]
# 定义方块类
class Shape:
def __init__(self, x, y):
self.x = x
self.y = y
self.type = random.randint(0, len(shapes) - 1)
self.color = colors[self.type]
self.shape = shapes[self.type]
self.rotation = 0
# 绘制方块
def draw(self, surface):
for r in range(len(self.shape)):
for c in range(len(self.shape[0])):
if self.shape[r][c] > 0:
pygame.draw.rect(surface, self.color, (self.x + c * block_size, self.y + r * block_size, block_size, block_size), 0)
# 移动方块
def move(self, dx, dy):
self.x += dx
self.y += dy
# 旋转方块
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
self.shape = shapes[self.type][self.rotation]
# 判断方块是否在游戏区域内
def is_valid(self):
for r in range(len(self.shape)):
for c in range(len(self.shape[0])):
if self.shape[r][c] > 0:
if self.x + c < 0 or self.x + c >= cols or self.y + r < 0 or self.y + r >= rows or board[self.y + r][self.x + c] > 0:
return False
return True
# 将方块固定在游戏区域中
def fix(self):
for r in range(len(self.shape)):
for c in range(len(self.shape[0])):
if self.shape[r][c] > 0:
board[self.y + r][self.x + c] = self.type + 1
# 定义游戏结束函数
def game_over():
font = pygame.font.SysFont("Arial", 60)
text = font.render("Game Over", True, red)
screen.blit(text, (width / 2 - text.get_width() / 2, height / 2 - text.get_height() / 2))
pygame.display.flip()
pygame.time.wait(2000)
pygame.quit()
sys.exit()
# 游戏主循环
fps = 5
clock = pygame.time.Clock()
current_shape = Shape(3 * block_size, 0)
next_shape = Shape(width + 3 * block_size, 0)
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_shape.move(-block_size, 0)
if not current_shape.is_valid():
current_shape.move(block_size, 0)
elif event.key == pygame.K_RIGHT:
current_shape.move(block_size, 0)
if not current_shape.is_valid():
current_shape.move(-block_size, 0)
elif event.key == pygame.K_UP:
current_shape.rotate()
if not current_shape.is_valid():
current_shape.rotate()
elif event.key == pygame.K_DOWN:
current_shape.move(0, block_size)
if not current_shape.is_valid():
current_shape.move(0, -block_size)
# 移动方块
current_shape.move(0, block_size)
if not current_shape.is_valid():
current_shape.move(0, -block_size)
current_shape.fix()
current_shape = next_shape
next_shape = Shape(width + 3 * block_size, 0)
if not current_shape.is_valid():
game_over()
# 绘制游戏区域
screen.fill(black)
for r in range(rows):
for c in range(cols):
pygame.draw.rect(screen, colors[board[r][c]], (c * block_size, r * block_size, block_size, block_size), 0)
# 绘制当前方块和下一个方块
current_shape.draw(screen)
next_shape.draw(screen)
pygame.display.flip()
clock.tick(fps)
```
这是一个比较简单的示例代码,还有很多可以优化的地方。如果您想了解更多关于 Pygame 的内容,可以参考 Pygame 官方文档。
相关推荐
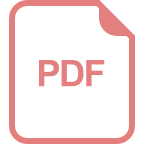








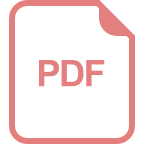
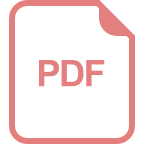
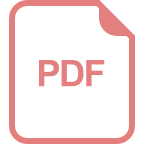
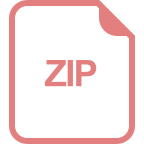
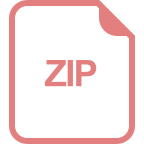
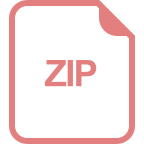