使用Java(4)创建一个Fraction类执行分数运算。要求如下: ①定义类的private成员变量:分子f1 和分母f2; ②提供构造方法,创建分数; ③提供两个分数相加的运算方法; ④提供两个分数相减的运算方法; ⑤提供两个分数相乘的运算方法; ⑥提供两个分数相除的运算方法; ⑦以a/b的形式打印Fraction数; ⑧以浮点数的形式打印Fraction数; ⑨主程序中创建分数,完成分数运算,输出结果
时间: 2024-01-21 10:19:50 浏览: 27
下面是一个简单的Fraction类的实现:
```java
public class Fraction {
private int f1; // 分子
private int f2; // 分母
// 构造方法
public Fraction(int f1, int f2) {
this.f1 = f1;
this.f2 = f2;
}
// 分数相加
public Fraction add(Fraction other) {
int newF1 = this.f1 * other.f2 + other.f1 * this.f2;
int newF2 = this.f2 * other.f2;
return new Fraction(newF1, newF2);
}
// 分数相减
public Fraction subtract(Fraction other) {
int newF1 = this.f1 * other.f2 - other.f1 * this.f2;
int newF2 = this.f2 * other.f2;
return new Fraction(newF1, newF2);
}
// 分数相乘
public Fraction multiply(Fraction other) {
int newF1 = this.f1 * other.f1;
int newF2 = this.f2 * other.f2;
return new Fraction(newF1, newF2);
}
// 分数相除
public Fraction divide(Fraction other) {
int newF1 = this.f1 * other.f2;
int newF2 = this.f2 * other.f1;
return new Fraction(newF1, newF2);
}
// 以a/b的形式打印分数
public String toString() {
return f1 + "/" + f2;
}
// 以浮点数的形式打印分数
public double toDouble() {
return (double) f1 / f2;
}
// 测试Fraction类
public static void main(String[] args) {
Fraction f1 = new Fraction(1, 2);
Fraction f2 = new Fraction(3, 4);
System.out.println(f1.add(f2)); // 5/4
System.out.println(f1.subtract(f2)); // -1/4
System.out.println(f1.multiply(f2)); // 3/8
System.out.println(f1.divide(f2)); // 2/3
System.out.println(f1.toString()); // 1/2
System.out.println(f1.toDouble()); // 0.5
}
}
```
在主方法中,我们创建了两个分数对象f1和f2,并对它们进行了加、减、乘、除等运算,并使用toString()方法以a/b的形式打印分数,使用toDouble()方法以浮点数的形式打印分数。
相关推荐
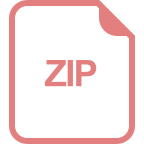
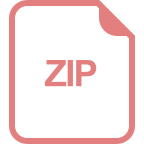
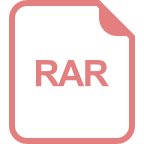












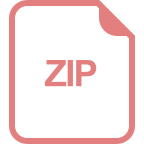
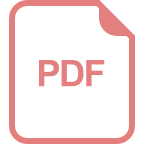