c语言打开wav处理数据后写入另一个wav例子
时间: 2023-12-04 17:03:10 浏览: 28
以下是一个示例代码,可以使用C语言打开一个WAV文件,处理数据,并将其写入另一个WAV文件:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
/* WAV文件头结构体 */
typedef struct {
char riff[4];
int size;
char wave[4];
char fmt[4];
int fmt_size;
short format;
short channels;
int sample_rate;
int byte_rate;
short block_align;
short bits_per_sample;
char data[4];
int data_size;
} WAV_HEADER;
int main() {
FILE* infile, * outfile;
WAV_HEADER header;
int data_bytes;
short* data_buffer;
int i;
/* 打开输入和输出文件 */
infile = fopen("input.wav", "rb");
outfile = fopen("output.wav", "wb");
/* 读取输入文件的WAV头信息 */
fread(&header, sizeof(WAV_HEADER), 1, infile);
/* 确认输入文件是PCM编码的 */
if (header.format != 1) {
printf("Error: Input file is not PCM encoded\n");
return -1;
}
/* 计算数据的字节数 */
data_bytes = header.data_size;
/* 分配输入数据缓冲区 */
data_buffer = (short*)malloc(data_bytes);
/* 读取输入文件的数据 */
fread(data_buffer, data_bytes, 1, infile);
/* 对数据进行处理(这里只是简单地将数据倒序)*/
for (i = 0; i < data_bytes / sizeof(short) / 2; i++) {
short temp = data_buffer[i];
data_buffer[i] = data_buffer[data_bytes / sizeof(short) - i - 1];
data_buffer[data_bytes / sizeof(short) - i - 1] = temp;
}
/* 写入输出文件的WAV头信息 */
fwrite(&header, sizeof(WAV_HEADER), 1, outfile);
/* 写入处理后的数据 */
fwrite(data_buffer, data_bytes, 1, outfile);
/* 关闭文件和释放内存 */
fclose(infile);
fclose(outfile);
free(data_buffer);
return 0;
}
```
这个示例程序打开了一个名为`input.wav`的WAV文件,并将数据倒序后写入了一个名为`output.wav`的新WAV文件。你可以根据自己的需求来修改该程序以实现其他处理方式。
相关推荐
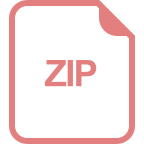














