c语言pcm转wav
时间: 2023-07-28 16:06:46 浏览: 79
将 PCM 格式的音频文件转换为 WAV 格式,可以使用以下 C 语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
typedef int16_t sample_t;
int main(int argc, char *argv[]) {
if (argc < 3) {
printf("Usage: pcm2wav <input file> <output file>\n");
return 1;
}
FILE *input_file = fopen(argv[1], "rb");
if (!input_file) {
printf("Failed to open input file: %s\n", argv[1]);
return 1;
}
FILE *output_file = fopen(argv[2], "wb");
if (!output_file) {
printf("Failed to open output file: %s\n", argv[2]);
fclose(input_file);
return 1;
}
// WAV 文件头
struct {
// RIFF 头
char riff_id[4]; // 固定为 "RIFF"
uint32_t riff_size; // 文件总长度 - 8
char wave_id[4]; // 固定为 "WAVE"
// fmt 子块
char fmt_id[4]; // 固定为 "fmt "
uint32_t fmt_size; // 固定为 16
uint16_t audio_format; // 编码格式,1 表示 PCM 格式
uint16_t num_channels; // 声道数,1 表示单声道,2 表示立体声
uint32_t sample_rate; // 采样率
uint32_t byte_rate; // 数据传输率,等于每秒采样数 × 每个样本的字节数
uint16_t block_align; // 每个采样块的字节数,等于每个样本的字节数 × 声道数
uint16_t bits_per_sample; // 每个样本的位数
// data 子块
char data_id[4]; // 固定为 "data"
uint32_t data_size; // 数据长度
} wav_header = {
.riff_id = "RIFF",
.wave_id = "WAVE",
.fmt_id = "fmt ",
.fmt_size = 16,
.audio_format = 1,
.num_channels = 1,
.sample_rate = 16000, // 假设采样率为 16000 Hz
.bits_per_sample = sizeof(sample_t) * 8,
.data_id = "data"
};
// 计算数据长度
fseek(input_file, 0, SEEK_END);
uint32_t data_size = ftell(input_file);
fseek(input_file, 0, SEEK_SET);
// 填写 WAV 文件头
wav_header.riff_size = 36 + data_size;
wav_header.byte_rate = wav_header.sample_rate * wav_header.num_channels * wav_header.bits_per_sample / 8;
wav_header.block_align = wav_header.num_channels * wav_header.bits_per_sample / 8;
wav_header.data_size = data_size;
// 写入 WAV 文件头
fwrite(&wav_header, sizeof(wav_header), 1, output_file);
// 复制数据
sample_t buffer[1024];
while (!feof(input_file)) {
size_t num_read = fread(buffer, sizeof(sample_t), sizeof(buffer) / sizeof(sample_t), input_file);
fwrite(buffer, sizeof(sample_t), num_read, output_file);
}
// 关闭文件
fclose(input_file);
fclose(output_file);
return 0;
}
```
你可以在命令行中使用以下命令来编译代码:
```
gcc pcm2wav.c -o pcm2wav
```
然后使用以下命令来将 PCM 文件转换为 WAV 文件:
```
./pcm2wav input.pcm output.wav
```
其中,`input.pcm` 是输入文件名,`output.wav` 是输出文件名。你需要将代码中的采样率、声道数等参数根据实际情况进行修改。
阅读全文
相关推荐
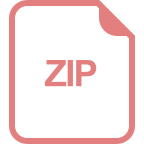
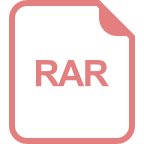
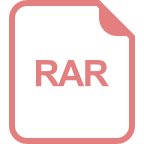
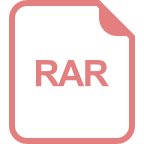
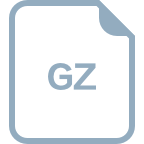
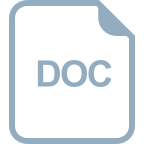
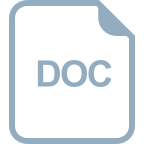


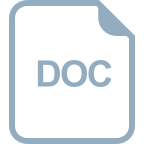
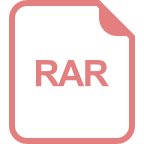
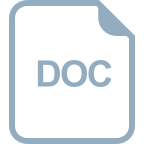



