wav转pcm使用c语言实现
时间: 2023-07-30 13:06:39 浏览: 102
要将wav文件转换为pcm格式,需要进行以下步骤:
1. 打开wav文件,读取文件头信息,获取音频格式、采样率、通道数等参数。
2. 读取wav文件中的音频数据,将其按照音频格式(如PCM、ADPCM、MP3等)进行解码。
3. 将解码后的音频数据保存为pcm格式,可以直接写入一个新的文件中。
以下是使用C语言实现wav转pcm的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// WAV文件头结构
typedef struct {
char riff[4]; // "RIFF"
int32_t size; // 文件长度
char wave[4]; // "WAVE"
char fmt[4]; // "fmt "
int32_t fmt_size; // fmt块长度
int16_t format; // 音频格式
int16_t channels; // 声道数
int32_t sample_rate; // 采样率
int32_t byte_rate; // 每秒数据量
int16_t block_align; // 数据块对齐单位
int16_t bits_per_sample; // 每个采样点的位数
char data[4]; // "data"
int32_t data_size; // 音频数据长度
} WavHeader;
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s input.wav output.pcm\n", argv[0]);
return -1;
}
// 打开输入文件
FILE *input_file = fopen(argv[1], "rb");
if (!input_file) {
printf("Failed to open input file %s\n", argv[1]);
return -1;
}
// 读取WAV文件头
WavHeader header;
fread(&header, sizeof(header), 1, input_file);
// 检查文件格式是否为WAV
if (strncmp(header.riff, "RIFF", 4) != 0 ||
strncmp(header.wave, "WAVE", 4) != 0 ||
strncmp(header.fmt, "fmt ", 4) != 0 ||
strncmp(header.data, "data", 4) != 0) {
printf("Invalid WAV file format\n");
return -1;
}
// 检查音频格式是否为PCM
if (header.format != 1) {
printf("Unsupported audio format\n");
return -1;
}
// 打开输出文件
FILE *output_file = fopen(argv[2], "wb");
if (!output_file) {
printf("Failed to open output file %s\n", argv[2]);
return -1;
}
// 读取音频数据
int16_t *buffer = (int16_t *)malloc(header.data_size);
fread(buffer, header.data_size, 1, input_file);
// 写入音频数据到PCM文件
fwrite(buffer, header.data_size, 1, output_file);
// 关闭文件
fclose(input_file);
fclose(output_file);
free(buffer);
return 0;
}
```
注意,这里简化了处理过程,并没有处理所有可能的wav文件格式,只是处理了一种比较常见的PCM格式。如果需要处理其他格式的音频文件,需要根据具体情况进行修改。
相关推荐
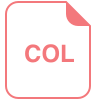
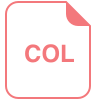
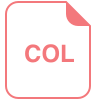











