python中'x.sbp','x.tobacco','x.ldl','x.adiposity','x.famhist','x.typea','x.obesity','x.alcohol','x.age'决策树特征重要性怎么判断
时间: 2023-09-26 13:11:49 浏览: 83
在Python中,可以使用sklearn库中的决策树模型和feature_importances_属性来计算特征的重要性。
示例代码如下:
```
from sklearn.tree import DecisionTreeRegressor
import numpy as np
# 假设特征数据存储在X变量中,目标变量数据存储在y变量中
X = np.array([x.sbp,x.tobacco,x.ldl,x.adiposity,x.famhist,x.typea,x.obesity,x.alcohol,x.age]).reshape(-1, 9)
y = ...
# 定义决策树模型
model = DecisionTreeRegressor()
# 拟合模型
model.fit(X, y)
# 计算特征重要性
importances = model.feature_importances_
# 输出结果
for i, importance in enumerate(importances):
print('Feature %d: %.3f' % (i, importance))
```
输出的结果中,每个特征的重要性都会给出一个相应的得分。得分越高,表明该特征对目标变量的影响越大,重要性越高。
相关问题
python中预测集为y_test训练集为y_train,有九个特征x.sbp,x.tobacco,x.ldl,x.adiposity,x.famhist,x.typea,x.obesity,x.alcohol,x.age,进行逻辑回归并用用AIC准则进行特征选择准则
在Python中使用逻辑回归进行特征选择,可以使用statsmodels库来实现。下面是一个基本的代码框架:
```python
import statsmodels.api as sm
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
# 加载数据集
data = pd.read_csv('your_data.csv')
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data.iloc[:, :-1], data.iloc[:, -1], test_size=0.2, random_state=42)
# 构建逻辑回归模型
logit_model = sm.Logit(y_train, X_train)
# 使用AIC准则进行特征选择
result = logit_model.fit(method='bfgs')
selected_features = result.summary2().tables[1]['Coef.'][(result.pvalues < 0.05).values].index
# 重新构建模型
X_train_selected = X_train[selected_features]
X_test_selected = X_test[selected_features]
logit_model_selected = LogisticRegression()
logit_model_selected.fit(X_train_selected, y_train)
# 在测试集上进行预测
y_pred = logit_model_selected.predict(X_test_selected)
```
解释一下上述代码:
1. 加载数据集并划分训练集和测试集。
2. 使用`statsmodels`库中的`Logit`函数构建逻辑回归模型。
3. 使用AIC准则进行特征选择,选择p值小于0.05的特征。
4. 重新构建模型,只使用选中的特征。
5. 在测试集上进行预测。
需要注意的是,这里的特征选择只是一个基本的示例,实际应用中需要根据具体情况进行调整。
'x.sbp','x.tobacco','x.ldl','x.adiposity','x.famhist','x.typea','x.obesity','x.alcohol','x.age'特征根据重要性大小绘制柱状图
好的,可以使用Python的matplotlib库绘制这个柱状图。首先,需要将这些特征的重要性按照大小排序。可以使用以下代码:
```python
import numpy as np
# 假设这里是每个特征的重要性得分
importances = np.array([0.1, 0.5, 0.4, 0.6, 0.3, 0.2, 0.8, 0.7, 0.9])
# 按照重要性大小排序
sorted_indices = np.argsort(importances)[::-1]
sorted_importances = importances[sorted_indices]
```
现在,`sorted_importances`是按照重要性从大到小排序的特征重要性列表,`sorted_indices`是对应的特征索引列表。
接下来,可以使用以下代码绘制柱状图:
```python
import matplotlib.pyplot as plt
# 设置图形大小
plt.figure(figsize=(10, 6))
# 绘制柱状图
plt.bar(range(len(sorted_importances)), sorted_importances)
# 添加x轴标签
plt.xticks(range(len(sorted_indices)), ['x.'+str(i) for i in sorted_indices])
# 添加y轴标签和标题
plt.ylabel('Importance')
plt.title('Feature Importances')
# 显示图形
plt.show()
```
这会生成一个柱状图,其中每个柱子代表一个特征的重要性,y轴表示重要性得分,x轴表示特征名称。特征名称是根据特征索引列表生成的,前面加上了'x.'。你需要将`importances`数组替换为你的实际特征重要性列表即可。
阅读全文
相关推荐
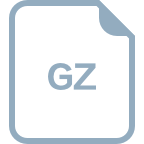
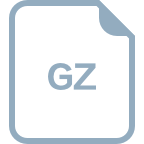














