用结构体和输入输出流编写一程序,允许从键盘输入任意多个英语单词,中间用空格分开,输入0表示输入结束。该程序可以统计同一个英语单词被输入几次,最后对英文单词按字典顺序输出,后面跟上该单词被输入的次数。
时间: 2023-09-12 20:05:03 浏览: 95
### 回答1:
以下是用 C 语言和结构体和输入输出流编写的程序,可以满足您的需求:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 1000
#define MAX_WORD_LENGTH 50
struct word_count {
char word[MAX_WORD_LENGTH];
int count;
};
int main() {
struct word_count words[MAX_WORDS];
int n = 0;
// 从键盘输入单词
char word[MAX_WORD_LENGTH];
scanf("%s", word);
while (strcmp(word, "0") != 0) {
// 检查单词是否已经存在
int index = -1;
for (int i = 0; i < n; i++) {
if (strcmp(words[i].word, word) == 0) {
index = i;
break;
}
}
// 如果单词不存在,添加新单词
if (index == -1) {
strcpy(words[n].word, word);
words[n].count = 1;
n++;
}
// 如果单词已经存在,增加计数器
else {
words[index].count++;
}
// 继续读取下一个单词
scanf("%s", word);
}
// 按字典顺序排序
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (strcmp(words[i].word, words[j].word) > 0) {
struct word_count temp = words[i];
words[i] = words[j];
words[j] = temp;
}
}
}
// 输出结果
for (int i = 0; i < n; i++) {
printf("%s %d\n", words[i].word, words[i].count);
}
return 0;
}
```
如果输入的单词数超过了 `MAX_WORDS`,或者单词的长度超过了 `MAX_WORD_LENGTH`,就会出现问题。如果您需要更严谨的程序,请根据实际情况进行修改。
### 回答2:
以下是使用结构体和输入输出流编写的一个程序,用于从键盘输入任意多个英语单词,并统计每个单词被输入的次数。最后,按照字典顺序输出每个单词及其输入次数。
```C++
#include<iostream>
#include<string>
#include<algorithm>
#include<map>
using namespace std;
struct Word {
string word;
int count;
};
bool compare(const Word& a, const Word& b) {
return a.word < b.word;
}
int main() {
string input;
map<string, int> wordCount;
cout << "请输入英语单词,以空格分隔,输入0表示结束:" << endl;
while (cin >> input && input != "0") {
++wordCount[input];
}
int uniqueWords = wordCount.size();
Word* words = new Word[uniqueWords];
int index = 0;
for (auto it = wordCount.begin(); it != wordCount.end(); ++it) {
words[index].word = it->first;
words[index].count = it->second;
++index;
}
sort(words, words + uniqueWords, compare);
cout << "按字典顺序输出单词及其输入次数:" << endl;
for (int i = 0; i < uniqueWords; ++i) {
cout << words[i].word << ": " << words[i].count << endl;
}
delete[] words;
return 0;
}
```
在这个程序中,我们使用了一个`map<string, int>`来存储每个单词及其对应的输入次数。我们首先从键盘上读取输入的单词,并通过递增`wordCount`中对应单词的输入次数来统计。然后,我们将每个单词和对应的次数保存在一个结构体数组`words`中。
接下来,我们使用`sort`函数对`words`数组进行排序,以按字典顺序输出。最后,我们通过遍历`words`数组,输出每个单词及其输入次数。
请注意,为了确保程序的正确运行,需要包含头文件`<iostream>`、`<string>`、`<algorithm>`和`<map>`。程序运行后,首先提示用户输入英语单词,以空格分隔,用户输入0来表示输入结束。然后,程序会按照字典顺序输出每个单词及其输入次数。
### 回答3:
首先,我们可以定义一个结构体来存储每个英语单词及其出现次数。结构体包含两个成员变量,一个是表示英语单词的字符串,另一个是表示出现次数的整数。
接下来,我们可以使用输入输出流来实现键盘输入和输出。可以使用cin来从键盘输入英语单词,直到输入0表示结束为止。同时,使用cout来输出排序后的英语单词及其出现次数。
下面是使用结构体和输入输出流编写的程序示例:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
struct WordCount {
string word;
int count;
};
bool compare(wordCount a, wordCount b) {
return a.word < b.word;
}
int main() {
const int maxSize = 100;
WordCount words[maxSize];
string input;
int numWords = 0;
cout << "请输入英语单词(输入0结束):" << endl;
cin >> input;
while (input != "0") {
// 检查输入的单词是否已存在
bool exists = false;
for (int i = 0; i < numWords; i++) {
if (input == words[i].word) {
words[i].count++;
exists = true;
break;
}
}
// 如果不存在,则将新的单词存入结构体数组中
if (!exists) {
words[numWords].word = input;
words[numWords].count = 1;
numWords++;
}
cin >> input;
}
// 对英语单词按字典顺序进行排序
sort(words, words + numWords, compare);
// 输出排序后的英语单词及其出现次数
cout << "按字典顺序输出英语单词及其出现次数:" << endl;
for (int i = 0; i < numWords; i++) {
cout << words[i].word << " " << words[i].count << endl;
}
return 0;
}
```
在该程序中,定义了一个结构体WordCount,包含两个成员变量:一个表示英语单词的字符串word,一个表示出现次数的整数count。使用了一个结构体数组来存储输入的英语单词及其出现次数。通过循环,判断输入的单词是否已经在结构体数组中存在,如果存在,则将该单词的出现次数加一,如果不存在,则将该单词及其出现次数存入结构体数组中。最后,使用sort函数对结构体数组进行排序,然后按字典顺序输出英语单词及其出现次数。
阅读全文
相关推荐
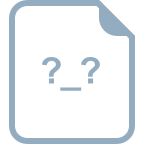
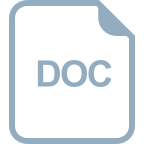











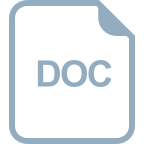
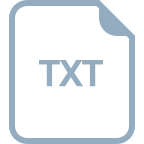