C++写一个检测蓝牙功能程序,检测蓝牙搜索数量是否达到在同级目录ini文本中设置的参数,并且把产生的测试log输出到同级目录文档中,其中蓝牙数量和文档名和配置档名字在配置档INI文件中设置键值对
时间: 2023-06-17 16:08:41 浏览: 58
以下是使用Winsock库实现的C++代码示例,用于检测蓝牙搜索数量是否达到在同级目录ini文本中设置的参数,并将测试log输出到同级目录文档中。
首先,需要在代码中包含Windows.h和Winsock2.h头文件,并链接ws2_32.lib库文件。
```cpp
#include <Windows.h>
#include <Winsock2.h>
#include <iostream>
#include <fstream>
#include <string>
#include <sstream>
#include <vector>
#include <map>
#pragma comment(lib, "ws2_32.lib")
```
然后,定义一个函数来读取INI文件中的配置项。
```cpp
std::map<std::string, std::string> readIniFile(std::string fileName) {
std::map<std::string, std::string> configMap;
std::ifstream file(fileName);
if (file.is_open()) {
std::string line;
std::string section = "";
while (getline(file, line)) {
if (line.empty()) {
continue;
}
if (line[0] == '[' && line[line.length() - 1] == ']') {
section = line.substr(1, line.length() - 2);
}
else {
std::stringstream ss(line);
std::string key, value;
getline(ss, key, '=');
getline(ss, value);
configMap[section + "." + key] = value;
}
}
file.close();
}
return configMap;
}
```
接着,定义一个函数来将log信息输出到文件中。
```cpp
void writeLogFile(std::string fileName, std::string logText) {
std::ofstream file(fileName, std::ios_base::app);
if (file.is_open()) {
file << logText << std::endl;
file.close();
}
}
```
最后,编写主函数来实现检测蓝牙搜索数量的功能。首先读取INI文件中的配置项,获取蓝牙数量和文档名字,并启动Winsock库。然后使用WSALookupServiceBegin函数开始搜索蓝牙设备,并在搜索到设备时统计数量。当蓝牙数量达到配置文件中设定的数量时,停止搜索并输出log信息到同级目录文档中。最后关闭Winsock库并退出程序。
```cpp
int main() {
std::map<std::string, std::string> config = readIniFile("config.ini");
int bluetoothCount = std::stoi(config["bluetooth.count"]);
std::string logFileName = config["log.filename"];
WSADATA wsaData;
int iResult = WSAStartup(MAKEWORD(2, 2), &wsaData);
if (iResult != 0) {
std::cout << "WSAStartup failed: " << iResult << std::endl;
return 1;
}
WSAQUERYSET service;
memset(&service, 0, sizeof(service));
service.dwSize = sizeof(service);
service.dwNameSpace = NS_BTH;
HANDLE lookupHandle = NULL;
iResult = WSALookupServiceBegin(&service, LUP_CONTAINERS, &lookupHandle);
if (iResult != 0) {
std::cout << "WSALookupServiceBegin failed: " << iResult << std::endl;
WSACleanup();
return 1;
}
int count = 0;
while (count < bluetoothCount) {
WSAQUERYSET result;
memset(&result, 0, sizeof(result));
result.dwSize = sizeof(result);
iResult = WSALookupServiceNext(lookupHandle, LUP_RETURN_NAME | LUP_RETURN_ADDR, &result);
if (iResult != 0) {
break;
}
count++;
}
WSALookupServiceEnd(lookupHandle);
WSACleanup();
if (count >= bluetoothCount) {
std::string logText = "Bluetooth count is " + std::to_string(count) + ", reached the target count of " + std::to_string(bluetoothCount);
writeLogFile(logFileName, logText);
}
else {
std::string logText = "Bluetooth count is " + std::to_string(count) + ", did not reach the target count of " + std::to_string(bluetoothCount);
writeLogFile(logFileName, logText);
}
return 0;
}
```
这样,我们就实现了一个简单的检测蓝牙搜索数量的程序,并将测试log输出到同级目录文档中。
相关推荐
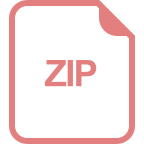
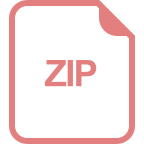
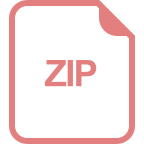














