直接迭代法求解非线性方程组c++
时间: 2024-09-25 21:07:21 浏览: 39
直接迭代法是一种数值方法,用于求解非线性方程组。在C++中,可以使用这种算法通过循环迭代的方式逼近每个方程的根。以下是使用固定点迭代法的一个简单示例:
```cpp
#include <iostream>
#include <vector>
// 假设我们有如下的非线性函数
std::vector<double> nonlinear_equations(const std::vector<double>& x) {
std::vector<double> f(x.size());
// 这里替换为实际的非线性方程表达式
for (size_t i = 0; i < x.size(); ++i) {
f[i] = x[i] * x[i] - 1; // 示例:x^2 - 1 = 0
}
return f;
}
// 使用牛顿法作为迭代器,这是一种常见的直接法
void fixed_point_iteration(double* x_start, double tolerance, size_t max_iterations) {
const auto n = x_start->size();
for (size_t iteration = 0; iteration < max_iterations && check_convergence(*x_start, tolerance); ++iteration) {
std::vector<double> current(x_start, x_start + n);
std::vector<double> next(n);
// 对每个方程求导并计算Jacobian矩阵
for (size_t j = 0; j < n; ++j) {
for (size_t k = 0; k < n; ++k) {
if (j == k) {
next[j] = current[j] - nonlinear_equations(current)[j] / current[j];
} else {
next[j] -= nonlinear_equations(current)[j] * (1.0 / current[k]);
}
}
}
// 更新x值
for (size_t i = 0; i < n; ++i) {
x_start[i] = next[i];
}
}
}
bool check_convergence(const std::vector<double>& x, double tolerance) {
double max_difference = 0.0;
for (double val : x) {
max_difference = std::max(max_difference, std::abs(val));
}
return max_difference < tolerance;
}
int main() {
double initial_guess[] = {1.5}; // 初始化猜测值
double tolerance = 1e-6;
size_t max_iterations = 1000;
fixed_point_iteration(initial_guess, tolerance, max_iterations);
std::cout << "Approximate solution: ";
for (const auto& value : initial_guess) {
std::cout << value << " ";
}
std::cout << "\n";
阅读全文
相关推荐
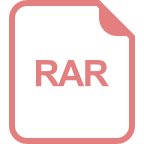
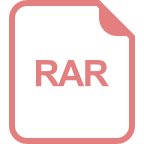
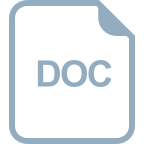
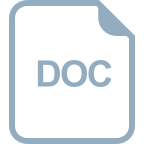
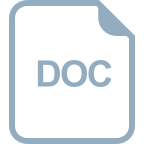
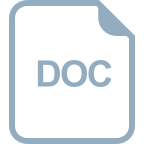
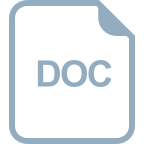
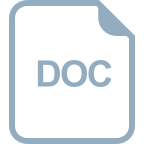

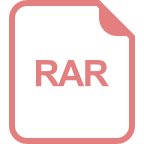
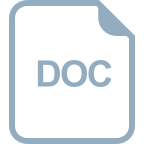
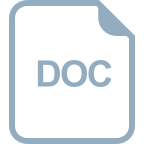

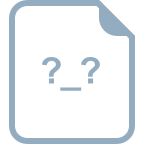
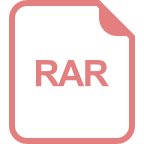
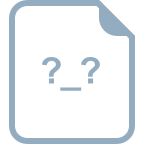
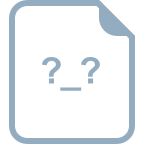

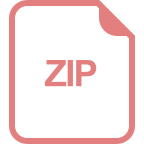