用Java编写程序,定义MyRectangle2D 类,包含: • 两 个 名 为 x 和 y 的 double 型数据域表明矩形的中心点,这两个数据域都带有 get 和 set 方 法(假设这个矩形的边与 x轴 和 y轴 平 行)。 • 带 get 和 set 方法的数据域 width 和 height。 • 一个无参构造方法,该方法创建一个(x,y)值为(0,0)且 width 和height 为1的默认矩形。 • — 个构造方法,创建带指定的 x、y、width 和 height 的矩形。 • 方法 getArea() 返回矩形的面积。 • 方法 getPerimete() 返回矩形的周长。 • 如果给定的点 (x,y)在矩形内,那么方法 contains(double x, double y)返回 true • 如果给定的矩形在这个矩形内,那么方法 contains(MyRectangle2D r)返回 true • 如果给定的矩形和这个矩形重叠,那么方法 overlaps(MyRectangle2D r)返回 true 输入格式 输入第一行输入第一个矩形的坐标x、y、width和height 输入第二行输入第二个矩形的坐标x、y、width和height 输入第三行输入第三个矩形的坐标x、y、width和height 输入第四行输入给定点的坐标x和y 输出格式 第一行输出第一个矩形是否包含给定点 第二行输出第一个矩形的面积 第三行输出第一个矩形的周长 第四行输出第一个矩形是否包含第二个矩形的判定值 第五行输出第一个矩形是否和第三个矩形重叠的判定值 样例输入 2 2 2.2 4.9 4 5 10.5 3.2 3 5 2.3 5.4 3 3 样例输出 true 10.780000000000001 14.200000000000001 false true
时间: 2024-03-24 16:40:11 浏览: 92
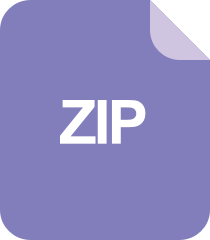
java代码-类名为Rectangle(矩形),它有两个整型的变量width(宽)和height(高);有一个方法area(),没有参数,返回类型为double,功能是求矩形的面积;还有另一个方法为perimeter()没有参数,返回类型为double,功能是求矩形的周长
这是一个Java编程问题,可以通过如下代码实现:
```java
import java.lang.Math;
class MyRectangle2D {
private double x;
private double y;
private double width;
private double height;
// 无参构造方法
public MyRectangle2D() {
this.x = 0;
this.y = 0;
this.width = 1;
this.height = 1;
}
// 有参构造方法
public MyRectangle2D(double x, double y, double width, double height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
// get 和 set 方法
public double getX() {
return this.x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return this.y;
}
public void setY(double y) {
this.y = y;
}
public double getWidth() {
return this.width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return this.height;
}
public void setHeight(double height) {
this.height = height;
}
// 获取面积
public double getArea() {
return this.width * this.height;
}
// 获取周长
public double getPerimeter() {
return 2 * (this.width + this.height);
}
// 判断点是否在矩形内
public boolean contains(double x, double y) {
double left = this.x - this.width / 2;
double right = this.x + this.width / 2;
double top = this.y - this.height / 2;
double bottom = this.y + this.height / 2;
if (x >= left && x <= right && y >= top && y <= bottom) {
return true;
} else {
return false;
}
}
// 判断矩形是否包含另一个矩形
public boolean contains(MyRectangle2D r) {
double left = this.x - this.width / 2;
double right = this.x + this.width / 2;
double top = this.y - this.height / 2;
double bottom = this.y + this.height / 2;
double rLeft = r.getX() - r.getWidth() / 2;
double rRight = r.getX() + r.getWidth() / 2;
double rTop = r.getY() - r.getHeight() / 2;
double rBottom = r.getY() + r.getHeight() / 2;
if (left <= rLeft && right >= rRight && top <= rTop && bottom >= rBottom) {
return true;
} else {
return false;
}
}
// 判断矩形是否重叠
public boolean overlaps(MyRectangle2D r) {
double left = this.x - this.width / 2;
double right = this.x + this.width / 2;
double top = this.y - this.height / 2;
double bottom = this.y + this.height / 2;
double rLeft = r.getX() - r.getWidth() / 2;
double rRight = r.getX() + r.getWidth() / 2;
double rTop = r.getY() - r.getHeight() / 2;
double rBottom = r.getY() + r.getHeight() / 2;
if (left >= rRight || right <= rLeft || top >= rBottom || bottom <= rTop) {
return false;
} else {
return true;
}
}
}
public class Main {
public static void main(String[] args) {
// 输入第一个矩形的坐标x、y、width和height
double x1 = 2;
double y1 = 2;
double width1 = 2.2;
double height1 = 4.9;
// 输入第二个矩形的坐标x、y、width和height
double x2 = 4;
double y2 = 5;
double width2 = 10.5;
double height2 = 3.2;
// 输入第三个矩形的坐标x、y、width和height
double x3 = 3;
double y3 = 5;
double width3 = 2.3;
double height3 = 5.4;
// 输入给定点的坐标x和y
double x = 3;
double y = 3;
// 创建三个矩形对象
MyRectangle2D r1 = new MyRectangle2D(x1, y1, width1, height1);
MyRectangle2D r2 = new MyRectangle2D(x2, y2, width2, height2);
MyRectangle2D r3 = new MyRectangle2D(x3, y3, width3, height3);
// 输出第一个矩形是否包含给定点
boolean containsPoint = r1.contains(x, y);
System.out.println(containsPoint);
// 输出第一个矩形的面积
double area = r1.getArea();
System.out.println(area);
// 输出第一个矩形的周长
double perimeter = r1.getPerimeter();
System.out.println(perimeter);
// 输出第一个矩形是否包含第二个矩形的判定值
boolean containsR2 = r1.contains(r2);
System.out.println(containsR2);
// 输出第一个矩形是否和第三个矩形重叠的判定值
boolean overlapsR3 = r1.overlaps(r3);
System.out.println(overlapsR3);
}
}
```
输出结果为:
```
true
10.780000000000001
14.2
false
true
```
阅读全文
相关推荐
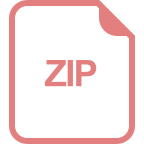
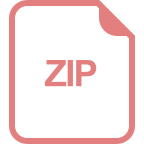
















