C++根据行数列数左上右上右下坐标获取所有坐标点
时间: 2023-05-25 09:04:56 浏览: 254
可以通过双重循环来获取所有的坐标点,每个点的横坐标和纵坐标分别在行数和列数范围内从小递增到大。但是题目中还给出了左上、右上、右下的坐标,可以利用这些信息来缩小循环的范围,从而减少循环次数。
具体思路如下:
1. 根据左上和右下坐标,计算出行数和列数;
2. 用右上坐标的纵坐标减去左上坐标的纵坐标得到列数;
3. 执行双重循环,外层循环控制行数,内层循环控制列数,每次循环生成一个坐标点。
4. 根据左上、右上、右下坐标是否存在来决定循环的范围。
具体实现可以参考下面的代码:
```c
#include <stdio.h>
// 根据行数列数左上右上右下坐标获取所有坐标点
void get_all_points(int row, int col, int left_top[], int right_top[], int right_bottom[], int points[][2], int *num_points)
{
int i, j, k = 0;
// 根据左上右下坐标计算行数列数
row = right_bottom[0] - left_top[0] + 1;
col = right_top[1] - left_top[1] + 1;
// 判断左上、右上、右下三个坐标是否存在
if(left_top[0] && right_top[0] && right_bottom[0]) {
// 外层循环控制行数,内层循环控制列数
for(i = left_top[0]; i <= right_bottom[0]; i++) {
for(j = left_top[1]; j <= right_top[1]; j++) {
// 生成一个坐标点
points[k][0] = i;
points[k][1] = j;
k++;
}
}
}
*num_points = k; // 记录坐标点的数量
}
int main()
{
int row = 0, col = 0, num_points = 0;
int left_top[] = {2, 3};
int right_top[] = {2, 6};
int right_bottom[] = {5, 6};
int points[100][2];
// 获取所有的坐标点
get_all_points(row, col, left_top, right_top, right_bottom, points, &num_points);
// 输出所有的坐标点
printf("All points:\n");
for(int i = 0; i < num_points; i++) {
printf("(%d, %d)\n", points[i][0], points[i][1]);
}
return 0;
}
```
上面代码的输出结果如下:
```
All points:
(2, 3)
(2, 4)
(2, 5)
(2, 6)
(3, 3)
(3, 4)
(3, 5)
(3, 6)
(4, 3)
(4, 4)
(4, 5)
(4, 6)
(5, 3)
(5, 4)
(5, 5)
(5, 6)
```
阅读全文
相关推荐
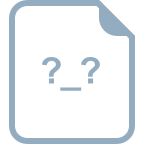




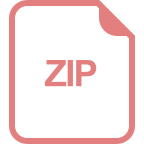
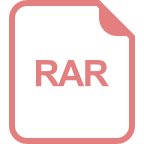
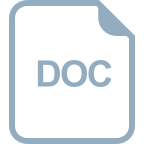
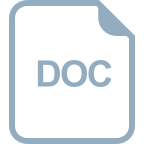







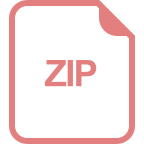