from tensorflow.keras.datasets import boston_housing 报错
时间: 2024-12-08 10:14:56 浏览: 34
当你运行 `from tensorflow.keras.datasets import boston_housing` 时收到错误,这通常是由于两个可能的问题:
1. TensorFlow Keras 库未正确安装或版本过旧:确保你已经安装了TensorFlow 2.x版本及以上,并且Keras是其内置的一部分。你可以通过运行 `pip install tensorflow>=2.0` 来检查或更新TensorFlow。
2. 数据集加载问题:从TensorFlow 2.1开始,Keras数据集模块的结构发生了变化。boston_housing现在位于`tf.keras.datasets`下的`load_data()`函数里,所以正确的导入应该是:
```python
from tensorflow.keras.datasets import boston_housing
(data_train, target_train), (data_test, target_test) = boston_housing.load_data()
```
如果已经安装了最新的TensorFlow并且仍然报错,可能是导入语句的细节有所更改,请查阅TensorFlow或Keras的官方文档,或者在网上搜索类似错误的解决方案。
相关问题
tensorflow采用keras序列模型,实现波士顿房价预测问题的建模与训练
好的,下面是用Keras序列模型实现波士顿房价预测问题的建模与训练的步骤:
1. 导入需要的库和数据集
```python
import numpy as np
from keras.datasets import boston_housing
from keras.models import Sequential
from keras.layers import Dense
# 导入波士顿房价数据集
(x_train, y_train), (x_test, y_test) = boston_housing.load_data()
```
2. 数据预处理
```python
# 标准化数据
mean = x_train.mean(axis=0)
std = x_train.std(axis=0)
x_train -= mean
x_train /= std
x_test -= mean
x_test /= std
# 将房价转化为 1000 美元为单位
y_train *= 1000
y_test *= 1000
```
3. 构建Keras序列模型
```python
model = Sequential()
model.add(Dense(64, activation='relu', input_shape=(13,)))
model.add(Dense(64, activation='relu'))
model.add(Dense(1))
```
4. 编译模型
```python
model.compile(loss='mse', optimizer='rmsprop', metrics=['mae'])
```
5. 训练模型
```python
history = model.fit(x_train, y_train, epochs=100, batch_size=16,
validation_split=0.2, verbose=0)
```
6. 评估模型
```python
test_mse_score, test_mae_score = model.evaluate(x_test, y_test)
print('Test MAE:', test_mae_score)
```
通过以上六步,我们可以使用Keras序列模型完成波士顿房价预测问题的建模与训练。
用tesnsorflow的keras实现attention_3d_block+TCN模型预测波士顿房价,过去5步预测未来1步
以下是使用TensorFlow的Keras实现带有注意力机制和TCN的模型来预测波士顿房价,并使用过去5步来预测未来1步的示例代码:
```python
import numpy as np
import pandas as pd
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense, Conv1D, GlobalMaxPooling1D, concatenate, Activation, Dropout
from tensorflow.keras.layers import Permute, Lambda, RepeatVector, Multiply, Add, Flatten
from tensorflow.keras import backend as K
# 读取数据并进行预处理
boston_housing = pd.read_csv('https://storage.googleapis.com/tf-datasets/tf_datasets/boston_housing.csv')
train_data = boston_housing.values[:, :-1]
train_target = boston_housing.values[:, -1]
train_data = (train_data - np.mean(train_data, axis=0)) / np.std(train_data, axis=0)
# 定义输入的形状
seq_len = 5
input_shape = (seq_len, train_data.shape[1])
input_layer = Input(shape=input_shape)
# 定义注意力机制
def attention_3d_block(inputs):
a = Permute((2, 1))(inputs)
a = Dense(seq_len, activation='softmax')(a)
a = Lambda(lambda x: K.mean(x, axis=1), name='dim_reduction')(a)
a = RepeatVector(train_data.shape[1])(a)
a_probs = Permute((2, 1), name='attention_vec')(a)
output_attention_mul = Multiply()([inputs, a_probs])
return output_attention_mul
# 定义TCN模型
def tcn_block(input_layer, n_filters, kernel_size, dilation_rate):
d = Conv1D(filters=n_filters, kernel_size=kernel_size, dilation_rate=dilation_rate, padding='causal')(input_layer)
d = Activation('relu')(d)
d = Dropout(0.2)(d)
return d
n_filters = 64
kernel_size = 2
dilation_rates = [2**i for i in range(3)]
dilated_layers = []
for dilation_rate in dilation_rates:
dilated_layers.append(tcn_block(input_layer, n_filters, kernel_size, dilation_rate))
concatenated_layers = concatenate(dilated_layers, axis=-1)
# 添加注意力机制
attention_layer = attention_3d_block(concatenated_layers)
# 全局最大池化
pooling_layer = GlobalMaxPooling1D()(attention_layer)
# 输出层
output_layer = Dense(1)(pooling_layer)
# 构建模型
model = Model(inputs=input_layer, outputs=output_layer)
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 训练模型
model.fit(train_data[:-seq_len-1], train_target[seq_len+1:], epochs=50, batch_size=32, validation_split=0.2)
# 预测未来1步
test_data = train_data[-seq_len:]
predicted_price = model.predict(np.expand_dims(test_data, axis=0))[0][0]
print("Predicted price for the next time step: ", predicted_price)
```
这个模型包含了一个注意力机制和TCN模块,可以用来预测波士顿房价。在这个例子中,我们使用过去5步来预测未来1步。
阅读全文
相关推荐
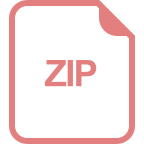
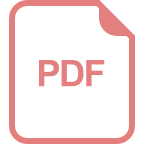
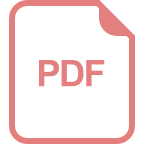













