def __init__(self, channels, hidden_channels=None, residual_activation=nn.Identity()): super(Resblock, self).__init__()是什么意思
时间: 2023-08-07 22:17:54 浏览: 48
这段代码是一个 PyTorch 的 Residual Block 实现,用于神经网络中的图像处理任务。其中:
- `__init__` 是 Python 类的构造函数,用于初始化类的属性。这里定义了 Resblock 类,它继承自 PyTorch 的 nn.Module 类。
- `channels` 是输入和输出的通道数。
- `hidden_channels` 是中间隐藏层的通道数,默认为输入和输出通道数相同。
- `residual_activation` 是残差连接中的激活函数,默认为恒等映射。
相关问题
class ResNetRGBD(nn.Module): def __init__(self, block, layers, num_classes=1000, zero_init_residual=False, groups=1, width_per_group=64, replace_stride_with_dilation=None, norm_layer=None): super(ResNetRGBD, self).__init__() if norm_layer is None: norm_layer = nn.BatchNorm2d self._norm_layer = norm_layer self.inplanes = 64 self.dilation = 1
这是一个 PyTorch 中的 ResNetRGBD 模型类的定义,它继承自 nn.Module 类。在初始化函数 `__init__` 中,它接受以下参数:
- `block`:ResNet 中残差块的类(比如 BasicBlock 或 Bottleneck)。
- `layers`:一个列表,表示每个残差块堆叠的数量。
- `num_classes`:输出类别的数量。
- `zero_init_residual`:是否使用零初始化残差块的权重。
- `groups`:分组卷积中的组数。
- `width_per_group`:每个组的通道数。
- `replace_stride_with_dilation`:是否使用空洞卷积代替步幅卷积来降低下采样率。
- `norm_layer`:归一化层的类型(比如 BatchNorm2d 或 GroupNorm)。
其中,`self._norm_layer` 表示使用的归一化层,`self.inplanes` 表示当前的通道数,`self.dilation` 表示当前的空洞率。
if residual: if in_dim != out_dim: self.res_fc = nn.Linear(in_dim, num_heads * out_dim, bias=False) nn.init.xavier_normal_(self.res_fc.weight.data, gain=1.414) else: self.res_fc = None
这段代码是一个条件语句,用于根据`residual`参数来添加或者不添加残差连接。
下面是对代码的解释:
- `if residual:`:这是一个条件语句,判断`residual`是否为真(非零)。
- `if in_dim != out_dim:`:如果输入维度`in_dim`不等于输出维度`out_dim`,则执行下面的代码块。
- `self.res_fc = nn.Linear(in_dim, num_heads * out_dim, bias=False)`:创建一个全连接层(`nn.Linear`)作为残差连接的线性变换。输入维度为`in_dim`,输出维度为`num_heads * out_dim`,没有偏置项。
- `nn.init.xavier_normal_(self.res_fc.weight.data, gain=1.414)`:对残差连接的线性变换的权重进行初始化,使用Xavier正态分布初始化方法,增益为1.414。
- `else:`:如果输入维度`in_dim`等于输出维度`out_dim`,则执行下面的代码块。
- `self.res_fc = None`:将残差连接设置为`None`,表示不使用残差连接。
通过这段代码,如果需要使用残差连接(`residual=True`),则根据输入和输出维度的关系创建一个线性变换层,并对其权重进行初始化。如果输入和输出维度相等,则不使用残差连接。残差连接可以帮助信息在网络中更好地传递,以提高模型的性能。
相关推荐
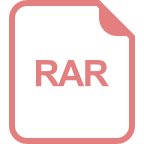
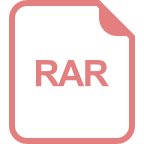













