ros::spinOnce()使用方法
时间: 2024-03-28 10:42:23 浏览: 17
`ros::spinOnce()`是ROS中一个非常重要的函数,其作用是使ROS可以处理当前已经接收到的所有回调函数。通常情况下,`ros::spinOnce()`会被放置在ROS节点的主循环中,以确保节点能够及时响应ROS系统的各种事件。
下面是`ros::spinOnce()`的使用方法:
1. 在ROS节点的主循环中,通过调用`ros::spinOnce()`来执行当前已经接收到的所有回调函数,例如:
```cpp
while (ros::ok())
{
ros::spinOnce();
// 进行其他的操作
// ...
}
```
2. 在一个回调函数中,如果需要在ROS系统中进行其他的操作,可以通过调用`ros::spinOnce()`来处理当前已经接收到的所有回调函数,例如:
```cpp
void myCallback(const my_msgs::MyMessage::ConstPtr& msg)
{
// 处理收到的消息
// ...
// 处理完消息后,调用ros::spinOnce()来执行当前已经接收到的所有回调函数
ros::spinOnce();
}
```
需要注意的是,如果在ROS节点的主循环中没有调用`ros::spinOnce()`,那么ROS系统将无法处理当前已经接收到的所有回调函数,从而导致节点无法及时响应ROS系统的各种事件。因此,在ROS节点的主循环中一定要记得调用`ros::spinOnce()`。
相关问题
ros::spinOnce
在ROS中,`ros::spinOnce()`是一个循环迭代函数,用于处理待处理的ROS消息。它通常与ROS Subscriber和Publisher一起使用,以确保ROS节点能够及时处理所有的消息。具体来说,`ros::spinOnce()`会在当前线程中处理所有待处理的ROS消息,然后返回,以便主程序可以继续执行其他任务。
在ROS节点的主循环中通常会调用`ros::spinOnce()`,以便ROS节点可以处理所有的ROS消息。例如,以下是一个ROS节点的简单主循环:
```cpp
ros::Rate loop_rate(10); // 定义循环频率为10Hz
while (ros::ok()) {
// 处理ROS消息
ros::spinOnce();
// 执行其他任务
// ...
// 控制循环频率
loop_rate.sleep();
}
```
在这个例子中,`ros::spinOnce()`被放置在主循环中,以便ROS节点能够在每次循环中处理所有待处理的ROS消息。在调用`ros::spinOnce()`之后,ROS节点将处理所有待处理的消息,包括所有订阅的消息和所有发布的消息。然后,ROS节点将返回,以便主程序可以执行其他任务。在本例中,我们使用`ros::Rate`对象控制循环的频率,以便ROS节点以10Hz的频率执行主循环。
总的来说,`ros::spinOnce()`是ROS中用于处理待处理的ROS消息的一个重要函数,通常与ROS Subscriber和Publisher一起使用,以确保ROS节点能够及时处理所有的消息。
ros::spinOnce();
`ros::spinOnce()`是ROS中一个非常重要的函数,它用于触发ROS回调函数的执行。在ROS节点中,通常会使用回调函数来处理订阅的消息或服务请求,因此需要定期调用`ros::spinOnce()`函数,以确保这些回调函数能够得到执行。
具体来说,`ros::spinOnce()`函数会检查ROS节点的订阅队列、服务队列和回调队列,如果有消息或服务请求到达,就会调用相应的回调函数进行处理。同时,`ros::spinOnce()`函数还会处理ROS节点的一些内部任务,例如发送和接收心跳消息等。
`ros::spinOnce()`函数通常在ROS节点的主循环中被调用,例如:
```
ros::Rate rate(20.0);
while (ros::ok())
{
// 处理一些任务
ros::spinOnce();
rate.sleep();
}
```
在这个例子中,`ros::spinOnce()`函数会被周期性地调用,以确保ROS节点能够及时处理消息和服务请求。需要注意的是,`ros::spinOnce()`函数不会阻塞程序,因此在其后面需要加上阻塞函数`rate.sleep()`,以控制程序的运行频率。
相关推荐
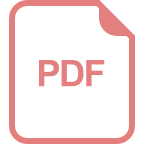
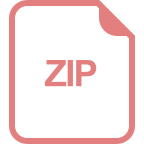
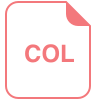
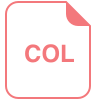
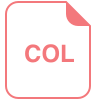
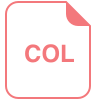
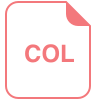







