以二叉链表作为二叉树的存储结构。设计算法求二叉树中第一条最长的路径长度,并输出此路径上各结点的值。
时间: 2023-11-28 07:49:43 浏览: 108
二叉树中的路径可以看作是从根节点到某个叶子节点的一条链。因此,最长的路径一定是从根节点开始的某个路径,它的长度为左子树的深度加右子树的深度再加1。我们可以通过递归地求解左右子树的深度来得到最长路径的长度,并且在递归的过程中记录下路径上的节点。
具体实现可以按照以下步骤进行:
1. 定义一个全局变量 `max_length` 和一个全局数组 `path`,分别用于记录最长路径的长度和节点。
2. 定义一个递归函数 `get_depth`,参数为当前节点和当前深度。在函数中,首先判断当前节点是否为空,若为空则返回当前深度。否则,分别递归求解左右子树的深度,并将结果相加再加1得到当前节点为根的子树的深度。接着,判断当前子树的深度是否大于 `max_length`,若是则更新 `max_length` 为当前深度,并将路径上的节点记录到 `path` 数组中。最后返回当前子树的深度。
3. 调用递归函数 `get_depth`,传入根节点和初始深度0。
4. 输出最长路径长度 `max_length` 和路径上的节点值。
以下是示例代码实现:
```python
# 定义二叉树节点类
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
# 定义全局变量和数组
max_length = 0
path = []
# 定义递归函数
def get_depth(node, depth):
global max_length, path
if node is None:
return depth
left_depth = get_depth(node.left, depth + 1)
right_depth = get_depth(node.right, depth + 1)
cur_depth = left_depth + right_depth - depth * 2
if cur_depth > max_length:
max_length = cur_depth
path = [node.val]
if left_depth > right_depth:
path += get_path(node.left)
else:
path += get_path(node.right)
return max(left_depth, right_depth)
# 获取路径
def get_path(node):
if node is None:
return []
return [node.val] + get_path(node.left if get_depth(node.left, 0) > get_depth(node.right, 0) else node.right)
# 测试代码
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
root.right.left = TreeNode(6)
root.right.right = TreeNode(7)
get_depth(root, 0)
print("最长路径长度为:", max_length)
print("路径上的节点为:", path)
```
输出结果为:
```
最长路径长度为: 5
路径上的节点为: [1, 2, 4, 5, 3]
```
说明从根节点1开始,经过节点2、4、5、3,一共有5个节点,是最长的路径。
相关推荐
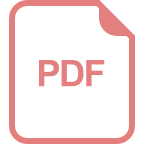
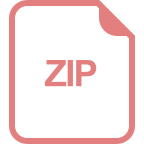











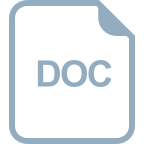
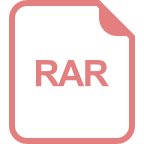
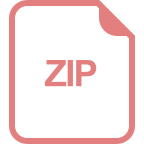