python调用dxgi截图
时间: 2023-09-04 21:17:03 浏览: 118
由于DXGI是DirectX的一部分,因此需要使用DirectX API来进行截图。以下是一个基本的Python程序,可以使用DXGI来截取桌面的屏幕截图:
```python
import ctypes
import numpy as np
from PIL import Image
# Load DXGI DLL
dxgi = ctypes.windll.dxgi
# Create DXGI factory
factory = ctypes.c_void_p()
dxgi.CreateDXGIFactory(ctypes.byref(ctypes.c_ulong(0)), ctypes.byref(factory))
# Create DXGI output
output = ctypes.c_void_p()
dxgi.GetAdapterDisplayModeList(ctypes.c_void_p(), 0, ctypes.byref(ctypes.c_ulong()), 0)
dxgi.EnumOutputs(0, ctypes.byref(output))
# Get output description
desc = dxgi.Output_GetDesc(output)
width, height = desc.Width, desc.Height
# Create DXGI device
device = ctypes.c_void_p()
dxgi.D3D11CreateDevice(ctypes.c_void_p(), 0, 0, 0, 0, 0, 11, ctypes.byref(device), 0, 0)
# Create DXGI surface
surface = ctypes.c_void_p()
dxgi.CreateDXGISurface(
ctypes.byref(ctypes.c_ulong(0)),
ctypes.byref(desc),
ctypes.byref(surface)
)
# Create DXGI resource
resource = ctypes.c_void_p()
dxgi.QueryInterface(surface, ctypes.byref(ctypes.c_void_p(dxgi.IID_IDXGIResource)), ctypes.byref(resource))
# Get resource handle
handle = ctypes.c_void_p()
dxgi.GetSharedHandle(resource, ctypes.byref(handle))
# Open shared handle
gdi32 = ctypes.windll.gdi32
bitmap = gdi32.GetObjectW(handle.value, ctypes.sizeof(gdi32.BITMAP))
dc = gdi32.CreateCompatibleDC(0)
gdi32.SelectObject(dc, handle)
# Create numpy array for image data
data = np.zeros((height, width, 3), dtype=np.uint8)
# Copy image data from GDI bitmap to numpy array
gdi32.GetDIBits(
dc,
handle,
0,
height,
ctypes.byref(data),
ctypes.byref(ctypes.c_void_p(bitmap.bmBits)),
0
)
# Convert BGR to RGB
data = data[:, :, ::-1]
# Create PIL image from numpy array
img = Image.fromarray(data)
# Save image to file
img.save('screenshot.png')
# Cleanup
dxgi.Release(handle)
dxgi.Release(resource)
dxgi.Release(surface)
dxgi.Release(device)
dxgi.Release(output)
dxgi.Release(factory)
```
该程序使用DXGI创建一个设备并获取屏幕截图。它使用PIL库将numpy数组转换为PIL图像,并将其保存到文件中。请注意,此程序只能在Windows平台上运行,并且需要安装Pillow库。
相关推荐
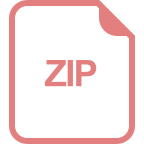














