不使用停用词(stop_words)的计数向量化器(CountVectorizer)怎么写python代码
时间: 2023-03-30 18:04:37 浏览: 118
可以使用sklearn库中的CountVectorizer类来实现不使用停用词的计数向量化器。具体的代码如下:
```python
from sklearn.feature_extraction.text import CountVectorizer
# 定义文本数据
text_data = ["I love coding in Python",
"Python is a great language",
"Java and Python are both popular programming languages"]
# 定义CountVectorizer对象
vectorizer = CountVectorizer(stop_words=None)
# 将文本数据转换为计数向量
count_vector = vectorizer.fit_transform(text_data)
# 输出计数向量
print(count_vector.toarray())
```
在上述代码中,我们首先定义了文本数据,然后创建了一个CountVectorizer对象,并将stop_words参数设置为None,表示不使用停用词。接着,我们使用fit_transform()方法将文本数据转换为计数向量,并使用toarray()方法将计数向量转换为数组形式进行输出。
相关问题
# 分词,并过滤停用词 stop_words <- stop_words$word comments_words <- comments_df_clean$content %>% unnest_tokens(word, content) %>% anti_join(stop_words),优化代码,使其可以读取字符型数据
可以使用以下代码来进行分词并过滤停用词,同时支持读取字符型数据:
```R
library(tidytext)
library(dplyr)
# 读取停用词表
stop_words <- data.frame(word = stopwords("en"))
# 读取数据并进行清洗
comments_df <- read.csv("comments.csv", stringsAsFactors = FALSE)
comments_df_clean <- comments_df %>%
filter(!is.na(content)) %>%
mutate(content = tolower(content)) %>%
mutate(content = gsub("[^[:alnum:] ]", " ", content)) %>%
mutate(content = gsub("\\s+", " ", content))
# 分词并过滤停用词
comments_words <- comments_df_clean$content %>%
unnest_tokens(word, content) %>%
anti_join(stop_words, by = "word")
```
其中,`read.csv()`函数用于读取CSV文件,`stringsAsFactors = FALSE`参数指定字符型数据不转换为因子型。`filter()`函数用于过滤空值,`tolower()`函数用于将文本转换为小写,`gsub()`函数用于替换不需要的字符,`unnest_tokens()`函数用于将文本分词,`anti_join()`函数用于过滤停用词。
利用停用词字典stop_words分别对文件夹中所有txt文档去停用词
好的,下面是一个基于 Python 的示例代码,可以实现利用停用词字典 `stop_words` 对文件夹中所有 txt 文档去停用词。你需要将 `stop_words` 字典替换为你自己的停用词字典,并将 `input_folder` 和 `output_folder` 分别替换为你的输入和输出文件夹路径。
```python
import os
import re
# 定义停用词字典
stop_words = {"is", "the", "of", "and", "a", "to", "in", "that", "it", "with", "for", "as", "on", "was", "at", "by", "an", "be", "this", "which", "from", "or", "were", "are", "you", "your", "we", "our"}
# 定义输入和输出文件夹路径
input_folder = "/path/to/input/folder"
output_folder = "/path/to/output/folder"
# 遍历文件夹中的所有 txt 文件
for filename in os.listdir(input_folder):
if filename.endswith(".txt"):
# 拼接文件路径
input_path = os.path.join(input_folder, filename)
output_path = os.path.join(output_folder, filename)
# 打开输入文件并读取内容
with open(input_path, "r", encoding="utf-8") as f:
text = f.read()
# 去除标点符号和数字
text = re.sub(r"[^\w\s]", "", text)
text = re.sub(r"\d+", "", text)
# 去除停用词
words = text.split()
words = [word for word in words if word not in stop_words]
text = " ".join(words)
# 将处理后的文本写入输出文件
with open(output_path, "w", encoding="utf-8") as f:
f.write(text)
```
这段代码将遍历指定的输入文件夹中的所有以 `.txt` 结尾的文件,对每个文件进行如下处理:
1. 打开文件并读取内容。
2. 去除标点符号和数字。
3. 去除停用词。
4. 将处理后的文本写入输出文件。
你需要根据自己的需求自行修改代码,比如替换停用词字典、修改输入和输出文件夹路径等。
阅读全文
相关推荐
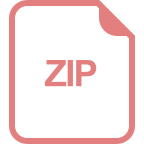
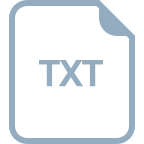
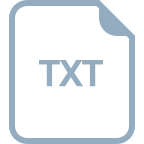












